diff --git a/CMakeLists.txt b/CMakeLists.txt
index daa4187..9b0022d 100644
--- a/CMakeLists.txt
+++ b/CMakeLists.txt
@@ -7,19 +7,25 @@ option(ENABLE_TSAN "Enable the thread data race sanitizer" OFF)
set(CMAKE_CXX_STANDARD 17)
+add_compile_options(-march=native)
+
+set(SQLITE_ORM_ENABLE_CXX_17 ON)
+
add_subdirectory(libs/blt)
add_subdirectory(libs/DPP-10.0.29)
+add_subdirectory(libs/sqlite_orm-1.8.2)
include_directories(include/)
file(GLOB_RECURSE PROJECT_BUILD_FILES "${CMAKE_CURRENT_SOURCE_DIR}/src/*.cpp")
add_executable(discord_bot ${PROJECT_BUILD_FILES})
-target_compile_options(discord_bot PRIVATE -Wall -Werror -Wpedantic -Wno-comment)
-target_link_options(discord_bot PRIVATE -Wall -Werror -Wpedantic -Wno-comment)
+target_compile_options(discord_bot PUBLIC -Wall -Wpedantic -Wno-comment -march=native)
+target_link_options(discord_bot PUBLIC -Wall -Wpedantic -Wno-comment)
target_link_libraries(discord_bot PUBLIC BLT)
target_link_libraries(discord_bot PUBLIC dpp)
+target_link_libraries(discord_bot PUBLIC sqlite_orm)
if (${ENABLE_ADDRSAN} MATCHES ON)
target_compile_options(discord_bot PRIVATE -fsanitize=address)
diff --git a/include/filemanager.h b/include/filemanager.h
new file mode 100644
index 0000000..ffab1d6
--- /dev/null
+++ b/include/filemanager.h
@@ -0,0 +1,145 @@
+#pragma once
+/*
+ * Copyright (C) 2024 Brett Terpstra
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#ifndef DISCORD_BOT_FILEMANAGER_H
+#define DISCORD_BOT_FILEMANAGER_H
+
+#include
+#include
+#include
+#include
+#include
+#include
+#include
+
+namespace db
+{
+ // path to archive_folder/raw/guildID/current_day/channelID(name)/
+ // in archive_folder/raw/guildID/
+ // (days)
+ // server_name.txt (changes in server name)
+ // migrations.txt (changes in channel name)
+ // channels.txt (deleted / create channels)
+
+ // in current_day:
+ // channelID
+
+ // message id: (username):
+ class msg_fs_manager
+ {
+ private:
+ std::fstream msg_file;
+ public:
+ explicit msg_fs_manager(const std::string& file): msg_file(file, std::ios::in | std::ios::out)
+ {}
+ };
+
+ class channel_fs_manager
+ {
+ private:
+ blt::u64 channelID;
+ HASHMAP msgs;
+ public:
+ explicit channel_fs_manager(blt::u64 channelID): channelID(channelID)
+ {}
+ };
+
+ class channel_flusher
+ {
+ private:
+ std::vector> channels_to_flush;
+ public:
+ void flush_channels(HASHMAP>& map);
+ };
+
+ class guild_fs_manager
+ {
+ private:
+ std::string archive_path;
+ std::string path;
+ blt::u64 guildID;
+ HASHMAP> channels;
+ dpp::cluster& bot;
+ channel_flusher flusher;
+ std::fstream f_server_name;
+ std::fstream f_migrations;
+ std::fstream f_channels;
+ std::atomic_int32_t complete{0};
+
+ void check_for_channel_updates();
+
+ void check_for_guild_updates();
+
+ public:
+ guild_fs_manager(std::string_view archive_path, std::string_view filePath, blt::u64 guildID, dpp::cluster& bot):
+ archive_path(archive_path), path(filePath), guildID(guildID), bot(bot),
+ f_server_name(this->archive_path + "server_name.txt", std::ios::out | std::ios::in | std::ios::app),
+ f_migrations(this->archive_path + "migrations.txt", std::ios::out | std::ios::in | std::ios::app),
+ f_channels(this->archive_path + "channels.txt", std::ios::out | std::ios::in | std::ios::app)
+ {}
+
+ void message_create(blt::u64 channel_id, blt::u64 msg_id, std::string_view content, std::string_view username,
+ std::string_view display_name, const std::vector& attachments);
+
+ void message_delete(blt::u64 channel_id, blt::u64 msg_id);
+
+ void message_bulk_delete(blt::u64 channel_id, const std::vector& message_ids)
+ {
+ for (const auto& v : message_ids)
+ message_delete(channel_id, format_as(v));
+ }
+
+ void message_update(blt::u64 channel_id, blt::u64 msg_id, std::string_view new_content);
+
+ void flush(std::string new_path);
+
+ void await_completions();
+ };
+
+ class fs_manager
+ {
+ private:
+ HASHMAP> guild_handlers;
+ dpp::cluster& bot;
+ std::string archive_path;
+ int current_day = 0;
+ std::atomic_bool is_flushing{false};
+
+ std::string create_path(blt::u64 guildID);
+
+ public:
+ explicit fs_manager(std::string_view archive_path, dpp::cluster& bot);
+
+ void create(blt::u64 guildID)
+ {
+ guild_handlers.insert({guildID, std::make_unique(archive_path, create_path(guildID), guildID, bot)});
+ }
+
+ void flush();
+
+ guild_fs_manager& operator[](blt::u64 guildID)
+ {
+ while (is_flushing)
+ {}
+ return *guild_handlers.at(guildID);
+ }
+ };
+
+}
+
+#endif //DISCORD_BOT_FILEMANAGER_H
diff --git a/libs/blt b/libs/blt
index 3845293..61d46de 160000
--- a/libs/blt
+++ b/libs/blt
@@ -1 +1 @@
-Subproject commit 384529333c0f46ef76dced3d78769250d3e227e6
+Subproject commit 61d46de5737b09d1eb1a6ef240f2af5e6ef2de71
diff --git a/libs/sqlite_orm-1.8.2/.clang-format b/libs/sqlite_orm-1.8.2/.clang-format
new file mode 100644
index 0000000..f4bc58f
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/.clang-format
@@ -0,0 +1,125 @@
+---
+Language: Cpp
+AccessModifierOffset: -2
+AlignAfterOpenBracket: Align
+AlignConsecutiveAssignments: false
+AlignConsecutiveDeclarations: false
+AlignEscapedNewlines: Right
+AlignOperands: true
+AlignTrailingComments: false
+AllowAllParametersOfDeclarationOnNextLine: false
+AllowShortLambdasOnASingleLine: Empty
+AllowAllArgumentsOnNextLine: false
+AllowAllConstructorInitializersOnNextLine: false
+AllowShortBlocksOnASingleLine: false
+AllowShortCaseLabelsOnASingleLine: false
+AllowShortFunctionsOnASingleLine: Empty
+AllowShortIfStatementsOnASingleLine: false
+AllowShortLoopsOnASingleLine: false
+AlwaysBreakAfterDefinitionReturnType: None
+AlwaysBreakAfterReturnType: None
+AlwaysBreakBeforeMultilineStrings: false
+AlwaysBreakTemplateDeclarations: Yes
+BinPackArguments: false
+BinPackParameters: false
+BraceWrapping:
+ AfterClass: false
+ AfterControlStatement: false
+ AfterEnum: false
+ AfterFunction: false
+ AfterNamespace: false
+ AfterObjCDeclaration: false
+ AfterStruct: false
+ AfterUnion: false
+ AfterExternBlock: false
+ BeforeCatch: false
+ BeforeElse: false
+ IndentBraces: false
+ SplitEmptyFunction: true
+ SplitEmptyRecord: true
+ SplitEmptyNamespace: true
+BreakBeforeBinaryOperators: None
+BreakBeforeBraces: Attach
+BreakBeforeInheritanceComma: false
+BreakInheritanceList: BeforeColon
+BreakBeforeTernaryOperators: true
+BreakConstructorInitializersBeforeComma: false
+BreakConstructorInitializers: AfterColon
+BreakAfterJavaFieldAnnotations: false
+BreakStringLiterals: true
+ColumnLimit: 120
+CommentPragmas: '^ IWYU pragma:'
+CompactNamespaces: false
+ConstructorInitializerAllOnOneLineOrOnePerLine: false
+ConstructorInitializerIndentWidth: 4
+ContinuationIndentWidth: 4
+Cpp11BracedListStyle: true
+DerivePointerAlignment: false
+DisableFormat: false
+ExperimentalAutoDetectBinPacking: false
+FixNamespaceComments: false
+ForEachMacros:
+ - foreach
+ - Q_FOREACH
+ - BOOST_FOREACH
+IncludeBlocks: Preserve
+IncludeCategories:
+ - Regex: '^"(llvm|llvm-c|clang|clang-c)/'
+ Priority: 2
+ - Regex: '^(<|"(gtest|gmock|isl|json)/)'
+ Priority: 3
+ - Regex: '.*'
+ Priority: 1
+IncludeIsMainRegex: '(Test)?$'
+IndentCaseLabels: true
+IndentPPDirectives: None
+IndentWidth: 4
+IndentWrappedFunctionNames: false
+JavaScriptQuotes: Leave
+JavaScriptWrapImports: true
+KeepEmptyLinesAtTheStartOfBlocks: true
+MacroBlockBegin: ''
+MacroBlockEnd: ''
+MaxEmptyLinesToKeep: 1
+NamespaceIndentation: All
+ObjCBinPackProtocolList: Auto
+ObjCBlockIndentWidth: 2
+ObjCSpaceAfterProperty: false
+ObjCSpaceBeforeProtocolList: true
+PenaltyBreakAssignment: 2
+PenaltyBreakBeforeFirstCallParameter: 19
+PenaltyBreakComment: 300
+PenaltyBreakFirstLessLess: 120
+PenaltyBreakString: 1000
+PenaltyBreakTemplateDeclaration: 10
+PenaltyExcessCharacter: 1000000
+PenaltyReturnTypeOnItsOwnLine: 60
+PointerAlignment: Left
+ReflowComments: false
+SortIncludes: false
+SortUsingDeclarations: true
+SpaceAfterCStyleCast: false
+SpaceAfterTemplateKeyword: false
+SpaceBeforeAssignmentOperators: true
+SpaceBeforeCpp11BracedList: false
+SpaceBeforeCtorInitializerColon: true
+SpaceBeforeInheritanceColon: true
+SpaceBeforeParens: Never
+SpaceBeforeRangeBasedForLoopColon: false
+SpaceInEmptyParentheses: false
+SpacesBeforeTrailingComments: 2
+SpacesInAngles: false
+SpacesInContainerLiterals: true
+SpacesInCStyleCastParentheses: false
+SpacesInParentheses: false
+SpacesInSquareBrackets: false
+Standard: Cpp11
+StatementMacros:
+ - __pragma
+ - _Pragma
+ - Q_UNUSED
+ - QT_REQUIRE_VERSION
+TabWidth: 8
+UseTab: Never
+...
+
diff --git a/libs/sqlite_orm-1.8.2/.github/FUNDING.yml b/libs/sqlite_orm-1.8.2/.github/FUNDING.yml
new file mode 100644
index 0000000..0ddfa7a
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/.github/FUNDING.yml
@@ -0,0 +1,2 @@
+github: fnc12
+custom: https://paypal.me/fnc12
diff --git a/libs/sqlite_orm-1.8.2/.github/workflows/clang-format-lint.yaml b/libs/sqlite_orm-1.8.2/.github/workflows/clang-format-lint.yaml
new file mode 100644
index 0000000..3916bbb
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/.github/workflows/clang-format-lint.yaml
@@ -0,0 +1,14 @@
+name: clang-format lint
+
+on: [push, pull_request]
+
+jobs:
+ build:
+ runs-on: ubuntu-latest
+
+ steps:
+ - uses: actions/checkout@v1
+ - name: clang-format lint
+ uses: DoozyX/clang-format-lint-action@v0.15
+ with:
+ clangFormatVersion: 15
diff --git a/libs/sqlite_orm-1.8.2/.gitignore b/libs/sqlite_orm-1.8.2/.gitignore
new file mode 100644
index 0000000..f5cff1a
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/.gitignore
@@ -0,0 +1,8 @@
+.DS_store
+examples/simple_neural_network.cpp
+
+cmake-build-debug/
+
+.idea/
+
+/compile
diff --git a/libs/sqlite_orm-1.8.2/.travis.yml b/libs/sqlite_orm-1.8.2/.travis.yml
new file mode 100644
index 0000000..d0a3685
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/.travis.yml
@@ -0,0 +1,157 @@
+# Defaults
+os: linux
+dist: focal
+
+matrix:
+ include:
+ - name: "[C++14] GCC-9"
+ addons:
+ apt:
+ sources:
+ - ubuntu-toolchain-r-test
+ packages:
+ - g++-9
+ - ninja-build
+ env:
+ - CC: gcc-9
+ - CXX: g++-9
+
+ - name: "[C++14] GCC-7"
+ addons:
+ apt:
+ sources:
+ - ubuntu-toolchain-r-test
+ packages:
+ - g++-7
+ - ninja-build
+ env:
+ - CC: gcc-7
+ - CXX: g++-7
+
+ - name: "[C++14] LLVM/Clang (Travis default)"
+ language: cpp
+ compiler: clang
+ addons:
+ apt:
+ packages:
+ - ninja-build
+ env:
+ - SQLITE_ORM_OMITS_CODECVT: ON
+
+ - name: "[C++14] AppleClang-10.0.1"
+ os: osx
+ osx_image: xcode10.2
+ language: cpp
+ env:
+ - SQLITE_ORM_OMITS_CODECVT: ON
+ addons:
+ homebrew:
+ packages:
+ - ninja
+ update: true
+
+ - name: "[C++14] LLVM/Clang (latest)"
+ os: osx
+ osx_image: xcode10.2
+ addons:
+ homebrew:
+ packages:
+ - llvm
+ - ninja
+ update: true
+ env:
+ - CPPFLAGS: "-I/usr/local/opt/llvm/include"
+ - LDFLAGS: "-L/usr/local/opt/llvm/lib -Wl,-rpath,/usr/local/opt/llvm/lib"
+ - CPATH: /usr/local/opt/llvm/include
+ - LIBRARY_PATH: /usr/local/opt/llvm/lib
+ - LD_LIBRARY_PATH: /usr/local/opt/llvm/lib
+ - CC: /usr/local/opt/llvm/bin/clang
+ - CXX: /usr/local/opt/llvm/bin/clang++
+ - SQLITE_ORM_OMITS_CODECVT: ON
+
+ - name: "[C++14] GCC-6"
+ os: osx
+ osx_image: xcode10.2
+ addons:
+ homebrew:
+ packages:
+ - gcc@6
+ - ninja
+ update: true
+ env:
+ - CC: gcc-6
+ - CXX: g++-6
+
+ - name: "[C++17] GCC-9"
+ addons:
+ apt:
+ sources:
+ - ubuntu-toolchain-r-test
+ packages:
+ - g++-9
+ - ninja-build
+ env:
+ - CC: gcc-9
+ - CXX: g++-9
+ - SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_17=ON"
+
+ - name: "[C++17] GCC-7"
+ addons:
+ apt:
+ sources:
+ - ubuntu-toolchain-r-test
+ packages:
+ - g++-7
+ - ninja-build
+ env:
+ - CC: gcc-7
+ - CXX: g++-7
+ - SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_17=ON"
+
+ - name: "[C++17] AppleClang-10.0.1"
+ os: osx
+ osx_image: xcode10.2
+ language: cpp
+ env:
+ - SQLITE_ORM_OMITS_CODECVT: ON
+ - SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_17=ON"
+ addons:
+ homebrew:
+ packages:
+ - ninja
+ update: true
+
+ - name: "[C++17] LLVM/Clang (latest)"
+ os: osx
+ osx_image: xcode10.2
+ addons:
+ homebrew:
+ packages:
+ - llvm
+ - ninja
+ update: true
+ env:
+ - CPPFLAGS: "-I/usr/local/opt/llvm/include"
+ - LDFLAGS: "-L/usr/local/opt/llvm/lib -Wl,-rpath,/usr/local/opt/llvm/lib"
+ - CPATH: /usr/local/opt/llvm/include
+ - LIBRARY_PATH: /usr/local/opt/llvm/lib
+ - LD_LIBRARY_PATH: /usr/local/opt/llvm/lib
+ - CC: /usr/local/opt/llvm/bin/clang
+ - CXX: /usr/local/opt/llvm/bin/clang++
+ - SQLITE_ORM_OMITS_CODECVT: ON
+ - SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_17=ON"
+
+before_install:
+ - if [[ ${TRAVIS_OS_NAME} == "osx" ]]; then export PATH="/usr/local/opt/coreutils/libexec/gnubin:$PATH"; fi
+
+# scripts to run before build
+before_script:
+ - if [[ "$CXX" == *"clang"* ]]; then clang --version ; fi
+ - cd ${TRAVIS_BUILD_DIR}
+ - mkdir compile && cd compile
+ - cmake -G Ninja -DCMAKE_BUILD_TYPE=Debug ${SQLITE_ORM_CXX_STANDARD} -DSQLITE_ORM_OMITS_CODECVT="${SQLITE_ORM_OMITS_CODECVT:OFF}" ..
+
+# build examples, and run tests (ie make & make test)
+script:
+ - cmake --build . --config Debug -- -k 10
+ - ctest --verbose --output-on-failure -C Debug -j $(nproc)
\ No newline at end of file
diff --git a/libs/sqlite_orm-1.8.2/CMakeLists.txt b/libs/sqlite_orm-1.8.2/CMakeLists.txt
new file mode 100644
index 0000000..a56294f
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/CMakeLists.txt
@@ -0,0 +1,93 @@
+# note: the minimum required version needs to go hand in hand with appveyor builds,
+# which is CMake 3.16 for the Visual Studio 2017 build worker image
+cmake_minimum_required (VERSION 3.16)
+
+# PACKAGE_VERSION is used by cpack scripts currently
+# Both sqlite_orm_VERSION and PACKAGE_VERSION should be the same for now
+
+set(sqlite_orm_VERSION "1.8.0")
+set(PACKAGE_VERSION ${sqlite_orm_VERSION})
+
+project("sqlite_orm" VERSION ${PACKAGE_VERSION})
+
+# Handling C++ standard version to use
+option(SQLITE_ORM_ENABLE_CXX_20 "Enable C++ 20" OFF)
+option(SQLITE_ORM_ENABLE_CXX_17 "Enable C++ 17" OFF)
+set(CMAKE_CXX_STANDARD_REQUIRED ON)
+if(SQLITE_ORM_ENABLE_CXX_20)
+ set(CMAKE_CXX_STANDARD 20)
+ message(STATUS "SQLITE_ORM: Build with C++20 features")
+elseif(SQLITE_ORM_ENABLE_CXX_17)
+ set(CMAKE_CXX_STANDARD 17)
+ message(STATUS "SQLITE_ORM: Build with C++17 features")
+else()
+ # fallback to C++14 if there is no special instruction
+ set(CMAKE_CXX_STANDARD 14)
+ message(STATUS "SQLITE_ORM: Build with C++14 features")
+endif()
+set(CMAKE_CXX_EXTENSIONS OFF)
+
+
+set(CMAKE_VERBOSE_MAKEFILE ON)
+
+message(STATUS "Configuring ${PROJECT_NAME} ${sqlite_orm_VERSION}")
+
+list(APPEND CMAKE_MODULE_PATH "${CMAKE_CURRENT_LIST_DIR}/cmake")
+
+include(CTest)
+
+### Dependencies
+add_subdirectory(dependencies)
+
+### Main Build Targets
+set(SqliteOrm_INCLUDE "${CMAKE_CURRENT_SOURCE_DIR}/include")
+add_library(sqlite_orm INTERFACE)
+add_library(sqlite_orm::sqlite_orm ALIAS sqlite_orm)
+
+find_package(SQLite3 REQUIRED)
+target_link_libraries(sqlite_orm INTERFACE SQLite::SQLite3)
+
+target_sources(sqlite_orm INTERFACE $)
+
+target_include_directories(sqlite_orm INTERFACE $)
+
+include(ucm)
+
+if (MSVC)
+ string(REGEX REPLACE "/RTC(su|[1su])" "" CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS}")
+ string(REGEX REPLACE "/RTC(su|[1su])" "" CMAKE_CXX_FLAGS_DEBUG "${CMAKE_CXX_FLAGS_DEBUG}")
+ string(REGEX REPLACE "/RTC(su|[1su])" "" CMAKE_CXX_FLAGS_RELEASE "${CMAKE_CXX_FLAGS_RELEASE}")
+ add_compile_options(/EHsc)
+ if (MSVC_VERSION GREATER_EQUAL 1914)
+ add_compile_options(/Zc:__cplusplus)
+ endif()
+ if (MSVC_VERSION GREATER_EQUAL 1910)
+ # VC 2017 issues a deprecation warning for `strncpy`
+ add_compile_definitions(_CRT_SECURE_NO_WARNINGS)
+ endif()
+ add_compile_options(/MP) # multi-processor compilation
+ if (CMAKE_CXX_STANDARD GREATER 14)
+ add_compile_definitions(_SILENCE_CXX17_CODECVT_HEADER_DEPRECATION_WARNING)
+ endif()
+
+ if ("${CMAKE_GENERATOR}" MATCHES "(Win64|x64)")
+ message(STATUS "Add /bigobj flag to compiler")
+ add_compile_options(/bigobj)
+ endif()
+endif()
+
+ucm_print_flags()
+message(STATUS "CMAKE_BUILD_TYPE: ${CMAKE_BUILD_TYPE}")
+
+# Tests
+if(CMAKE_PROJECT_NAME STREQUAL PROJECT_NAME AND BUILD_TESTING)
+ add_subdirectory(tests)
+endif()
+
+option(BUILD_EXAMPLES "Build code examples" OFF)
+if(CMAKE_PROJECT_NAME STREQUAL PROJECT_NAME AND BUILD_EXAMPLES)
+ add_subdirectory(examples)
+endif()
+
+### Packaging
+add_subdirectory(packaging)
diff --git a/libs/sqlite_orm-1.8.2/COMM-LICENSE b/libs/sqlite_orm-1.8.2/COMM-LICENSE
new file mode 100644
index 0000000..d64eaa5
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/COMM-LICENSE
@@ -0,0 +1,20 @@
+Copyright (c) 2012-2023 Eugene Zakharov and others
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/libs/sqlite_orm-1.8.2/CONTRIBUTING.md b/libs/sqlite_orm-1.8.2/CONTRIBUTING.md
new file mode 100644
index 0000000..b1baf53
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/CONTRIBUTING.md
@@ -0,0 +1,94 @@
+# How to Contribute #
+
+Thank you for your interest in contributing to the sqlite_orm project!
+
+## GitHub pull requests ##
+
+This is the preferred method of submitting changes. When you submit a pull request through github,
+it activates the continuous integration (CI) build systems at Appveyor and Travis to build your changes
+on a variety of Linux, Windows and MacOS configurations and run all the test suites. Follow these requirements
+for a successful pull request:
+
+ 1. All significant changes require a [github issue](https://github.com/fnc12/sqlite_orm/issues). Trivial changes such as fixing a typo or a compiler warning do not.
+
+ 1. The pull request title must begin with the github issue identifier if it has an associated issue, for example:
+
+ #9999 : an example pull request title
+
+ 1. Commit messages must be understandable in future by different developers and must be written in english language only:
+
+Instructions:
+
+ 1. Create a fork in your GitHub account of http://github.com/fnc12/sqlite_orm
+ 1. Clone the fork to your development system.
+ 1. Create a branch for your changes (best practice is following git flow pattern with issue number as branch name, e.g. feature/9999-some-feature or bugfix/9999-some-bug).
+ 1. Modify the source to include the improvement/bugfix, and:
+
+ * Remember to provide *tests* for all submitted changes!
+ * Use test-driven development (TDD): add a test that will isolate the bug *before* applying the change that fixes it.
+ * Verify that you follow current code style on sqlite_orm.
+ * [*optional*] Verify that your change works on other platforms by adding a GitHub service hook to [Travis CI](http://docs.travis-ci.com/user/getting-started/#Step-one%3A-Sign-in) and [AppVeyor](http://www.appveyor.com/docs). You can use this technique to run the sqlite_orm CI jobs in your account to check your changes before they are made public. Every GitHub pull request into sqlite_orm will run the full CI build and test suite on your changes.
+
+ 1. Commit and push changes to your branch (please use issue name and description as commit title, e.g. "make it perfect. (fixes #9999)").
+ 1. Use GitHub to create a pull request going from your branch to sqlite_orm:dev. Ensure that the github issue number is at the beginning of the title of your pull request.
+ 1. Wait for other contributors or committers to review your new addition, and for a CI build to complete.
+ 1. Wait for a owner or collaborators to commit your patch.
+
+## If you want to build the project locally ##
+
+See our detailed instructions on the [CMake README](https://github.com/fnc12/sqlite_orm#usage).
+
+## If you want to review open issues... ##
+
+ 1. Review the [GitHub Pull Request Backlog](https://github.com/fnc12/sqlite_orm/pulls). Code reviews are opened to all.
+
+## If you discovered a defect... ##
+
+ 1. Check to see if the issue is already in the [github issues](https://github.com/fnc12/sqlite_orm/issues).
+ 1. If not please create an issue describing the change you're proposing in the github issues page.
+ 1. Contribute your code changes using the GitHub pull request method:
+
+## GitHub recipes for Pull Requests ##
+
+Sometimes commmitters may ask you to take actions in your pull requests. Here are some recipes that will help you accomplish those requests. These examples assume you are working on github issue 9999. You should also be familiar with the [upstream](https://help.github.com/articles/syncing-a-fork/) repository concept.
+
+### Squash your changes ###
+
+If you have commits with adding code which is removed in a different commit within the same PR then please squash all commits to remove unnecessary add commits.
+
+1. Use the command ``git log`` to identify how many commits you made since you began.
+2. Use the command ``git rebase -i HEAD~N`` where N is the number of commits.
+3. Leave "pull" in the first line.
+4. Change all other lines from "pull" to "fixup".
+5. All your changes are now in a single commit.
+
+If you already have a pull request outstanding, you will need to do a "force push" to overwrite it since you changed your commit history:
+
+ git push -u origin feature/9999-make-perfect --force
+
+A more detailed walkthrough of a squash can be found at [Git Ready](http://gitready.com/advanced/2009/02/10/squashing-commits-with-rebase.html).
+
+### Rebase your pull request ###
+
+If your pull request has a conflict with dev, it needs to be rebased:
+
+ git checkout feature/9999-make-perfect
+ git rebase upstream dev
+ (resolve any conflicts, make sure it builds)
+ git push -u origin feature/9999-make-perfect --force
+
+### Fix a bad merge ###
+
+If your pull request contains commits that are not yours, then you should use the following technique to fix the bad merge in your branch:
+
+ git checkout dev
+ git pull upstream dev
+ git checkout -b feature/9999-make-perfect-take-2
+ git cherry-pick ...
+ (pick only your commits from your original pull request in ascending chronological order)
+ squash your changes to a single commit if there is more than one (see above)
+ git push -u origin feature/9999-make-perfect-take-2:feature/9999-make-perfect
+
+This procedure will apply only your commits in order to the current dev, then you will squash them to a single commit, and then you force push your local feature/9999-make-perfect-take-2 into remote feature/9999-make-perfect which represents your pull request, replacing all the commits with the new one.
+
+
diff --git a/libs/sqlite_orm-1.8.2/LICENSE b/libs/sqlite_orm-1.8.2/LICENSE
new file mode 100644
index 0000000..0ad25db
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/LICENSE
@@ -0,0 +1,661 @@
+ GNU AFFERO GENERAL PUBLIC LICENSE
+ Version 3, 19 November 2007
+
+ Copyright (C) 2007 Free Software Foundation, Inc.
+ Everyone is permitted to copy and distribute verbatim copies
+ of this license document, but changing it is not allowed.
+
+ Preamble
+
+ The GNU Affero General Public License is a free, copyleft license for
+software and other kinds of works, specifically designed to ensure
+cooperation with the community in the case of network server software.
+
+ The licenses for most software and other practical works are designed
+to take away your freedom to share and change the works. By contrast,
+our General Public Licenses are intended to guarantee your freedom to
+share and change all versions of a program--to make sure it remains free
+software for all its users.
+
+ When we speak of free software, we are referring to freedom, not
+price. Our General Public Licenses are designed to make sure that you
+have the freedom to distribute copies of free software (and charge for
+them if you wish), that you receive source code or can get it if you
+want it, that you can change the software or use pieces of it in new
+free programs, and that you know you can do these things.
+
+ Developers that use our General Public Licenses protect your rights
+with two steps: (1) assert copyright on the software, and (2) offer
+you this License which gives you legal permission to copy, distribute
+and/or modify the software.
+
+ A secondary benefit of defending all users' freedom is that
+improvements made in alternate versions of the program, if they
+receive widespread use, become available for other developers to
+incorporate. Many developers of free software are heartened and
+encouraged by the resulting cooperation. However, in the case of
+software used on network servers, this result may fail to come about.
+The GNU General Public License permits making a modified version and
+letting the public access it on a server without ever releasing its
+source code to the public.
+
+ The GNU Affero General Public License is designed specifically to
+ensure that, in such cases, the modified source code becomes available
+to the community. It requires the operator of a network server to
+provide the source code of the modified version running there to the
+users of that server. Therefore, public use of a modified version, on
+a publicly accessible server, gives the public access to the source
+code of the modified version.
+
+ An older license, called the Affero General Public License and
+published by Affero, was designed to accomplish similar goals. This is
+a different license, not a version of the Affero GPL, but Affero has
+released a new version of the Affero GPL which permits relicensing under
+this license.
+
+ The precise terms and conditions for copying, distribution and
+modification follow.
+
+ TERMS AND CONDITIONS
+
+ 0. Definitions.
+
+ "This License" refers to version 3 of the GNU Affero General Public License.
+
+ "Copyright" also means copyright-like laws that apply to other kinds of
+works, such as semiconductor masks.
+
+ "The Program" refers to any copyrightable work licensed under this
+License. Each licensee is addressed as "you". "Licensees" and
+"recipients" may be individuals or organizations.
+
+ To "modify" a work means to copy from or adapt all or part of the work
+in a fashion requiring copyright permission, other than the making of an
+exact copy. The resulting work is called a "modified version" of the
+earlier work or a work "based on" the earlier work.
+
+ A "covered work" means either the unmodified Program or a work based
+on the Program.
+
+ To "propagate" a work means to do anything with it that, without
+permission, would make you directly or secondarily liable for
+infringement under applicable copyright law, except executing it on a
+computer or modifying a private copy. Propagation includes copying,
+distribution (with or without modification), making available to the
+public, and in some countries other activities as well.
+
+ To "convey" a work means any kind of propagation that enables other
+parties to make or receive copies. Mere interaction with a user through
+a computer network, with no transfer of a copy, is not conveying.
+
+ An interactive user interface displays "Appropriate Legal Notices"
+to the extent that it includes a convenient and prominently visible
+feature that (1) displays an appropriate copyright notice, and (2)
+tells the user that there is no warranty for the work (except to the
+extent that warranties are provided), that licensees may convey the
+work under this License, and how to view a copy of this License. If
+the interface presents a list of user commands or options, such as a
+menu, a prominent item in the list meets this criterion.
+
+ 1. Source Code.
+
+ The "source code" for a work means the preferred form of the work
+for making modifications to it. "Object code" means any non-source
+form of a work.
+
+ A "Standard Interface" means an interface that either is an official
+standard defined by a recognized standards body, or, in the case of
+interfaces specified for a particular programming language, one that
+is widely used among developers working in that language.
+
+ The "System Libraries" of an executable work include anything, other
+than the work as a whole, that (a) is included in the normal form of
+packaging a Major Component, but which is not part of that Major
+Component, and (b) serves only to enable use of the work with that
+Major Component, or to implement a Standard Interface for which an
+implementation is available to the public in source code form. A
+"Major Component", in this context, means a major essential component
+(kernel, window system, and so on) of the specific operating system
+(if any) on which the executable work runs, or a compiler used to
+produce the work, or an object code interpreter used to run it.
+
+ The "Corresponding Source" for a work in object code form means all
+the source code needed to generate, install, and (for an executable
+work) run the object code and to modify the work, including scripts to
+control those activities. However, it does not include the work's
+System Libraries, or general-purpose tools or generally available free
+programs which are used unmodified in performing those activities but
+which are not part of the work. For example, Corresponding Source
+includes interface definition files associated with source files for
+the work, and the source code for shared libraries and dynamically
+linked subprograms that the work is specifically designed to require,
+such as by intimate data communication or control flow between those
+subprograms and other parts of the work.
+
+ The Corresponding Source need not include anything that users
+can regenerate automatically from other parts of the Corresponding
+Source.
+
+ The Corresponding Source for a work in source code form is that
+same work.
+
+ 2. Basic Permissions.
+
+ All rights granted under this License are granted for the term of
+copyright on the Program, and are irrevocable provided the stated
+conditions are met. This License explicitly affirms your unlimited
+permission to run the unmodified Program. The output from running a
+covered work is covered by this License only if the output, given its
+content, constitutes a covered work. This License acknowledges your
+rights of fair use or other equivalent, as provided by copyright law.
+
+ You may make, run and propagate covered works that you do not
+convey, without conditions so long as your license otherwise remains
+in force. You may convey covered works to others for the sole purpose
+of having them make modifications exclusively for you, or provide you
+with facilities for running those works, provided that you comply with
+the terms of this License in conveying all material for which you do
+not control copyright. Those thus making or running the covered works
+for you must do so exclusively on your behalf, under your direction
+and control, on terms that prohibit them from making any copies of
+your copyrighted material outside their relationship with you.
+
+ Conveying under any other circumstances is permitted solely under
+the conditions stated below. Sublicensing is not allowed; section 10
+makes it unnecessary.
+
+ 3. Protecting Users' Legal Rights From Anti-Circumvention Law.
+
+ No covered work shall be deemed part of an effective technological
+measure under any applicable law fulfilling obligations under article
+11 of the WIPO copyright treaty adopted on 20 December 1996, or
+similar laws prohibiting or restricting circumvention of such
+measures.
+
+ When you convey a covered work, you waive any legal power to forbid
+circumvention of technological measures to the extent such circumvention
+is effected by exercising rights under this License with respect to
+the covered work, and you disclaim any intention to limit operation or
+modification of the work as a means of enforcing, against the work's
+users, your or third parties' legal rights to forbid circumvention of
+technological measures.
+
+ 4. Conveying Verbatim Copies.
+
+ You may convey verbatim copies of the Program's source code as you
+receive it, in any medium, provided that you conspicuously and
+appropriately publish on each copy an appropriate copyright notice;
+keep intact all notices stating that this License and any
+non-permissive terms added in accord with section 7 apply to the code;
+keep intact all notices of the absence of any warranty; and give all
+recipients a copy of this License along with the Program.
+
+ You may charge any price or no price for each copy that you convey,
+and you may offer support or warranty protection for a fee.
+
+ 5. Conveying Modified Source Versions.
+
+ You may convey a work based on the Program, or the modifications to
+produce it from the Program, in the form of source code under the
+terms of section 4, provided that you also meet all of these conditions:
+
+ a) The work must carry prominent notices stating that you modified
+ it, and giving a relevant date.
+
+ b) The work must carry prominent notices stating that it is
+ released under this License and any conditions added under section
+ 7. This requirement modifies the requirement in section 4 to
+ "keep intact all notices".
+
+ c) You must license the entire work, as a whole, under this
+ License to anyone who comes into possession of a copy. This
+ License will therefore apply, along with any applicable section 7
+ additional terms, to the whole of the work, and all its parts,
+ regardless of how they are packaged. This License gives no
+ permission to license the work in any other way, but it does not
+ invalidate such permission if you have separately received it.
+
+ d) If the work has interactive user interfaces, each must display
+ Appropriate Legal Notices; however, if the Program has interactive
+ interfaces that do not display Appropriate Legal Notices, your
+ work need not make them do so.
+
+ A compilation of a covered work with other separate and independent
+works, which are not by their nature extensions of the covered work,
+and which are not combined with it such as to form a larger program,
+in or on a volume of a storage or distribution medium, is called an
+"aggregate" if the compilation and its resulting copyright are not
+used to limit the access or legal rights of the compilation's users
+beyond what the individual works permit. Inclusion of a covered work
+in an aggregate does not cause this License to apply to the other
+parts of the aggregate.
+
+ 6. Conveying Non-Source Forms.
+
+ You may convey a covered work in object code form under the terms
+of sections 4 and 5, provided that you also convey the
+machine-readable Corresponding Source under the terms of this License,
+in one of these ways:
+
+ a) Convey the object code in, or embodied in, a physical product
+ (including a physical distribution medium), accompanied by the
+ Corresponding Source fixed on a durable physical medium
+ customarily used for software interchange.
+
+ b) Convey the object code in, or embodied in, a physical product
+ (including a physical distribution medium), accompanied by a
+ written offer, valid for at least three years and valid for as
+ long as you offer spare parts or customer support for that product
+ model, to give anyone who possesses the object code either (1) a
+ copy of the Corresponding Source for all the software in the
+ product that is covered by this License, on a durable physical
+ medium customarily used for software interchange, for a price no
+ more than your reasonable cost of physically performing this
+ conveying of source, or (2) access to copy the
+ Corresponding Source from a network server at no charge.
+
+ c) Convey individual copies of the object code with a copy of the
+ written offer to provide the Corresponding Source. This
+ alternative is allowed only occasionally and noncommercially, and
+ only if you received the object code with such an offer, in accord
+ with subsection 6b.
+
+ d) Convey the object code by offering access from a designated
+ place (gratis or for a charge), and offer equivalent access to the
+ Corresponding Source in the same way through the same place at no
+ further charge. You need not require recipients to copy the
+ Corresponding Source along with the object code. If the place to
+ copy the object code is a network server, the Corresponding Source
+ may be on a different server (operated by you or a third party)
+ that supports equivalent copying facilities, provided you maintain
+ clear directions next to the object code saying where to find the
+ Corresponding Source. Regardless of what server hosts the
+ Corresponding Source, you remain obligated to ensure that it is
+ available for as long as needed to satisfy these requirements.
+
+ e) Convey the object code using peer-to-peer transmission, provided
+ you inform other peers where the object code and Corresponding
+ Source of the work are being offered to the general public at no
+ charge under subsection 6d.
+
+ A separable portion of the object code, whose source code is excluded
+from the Corresponding Source as a System Library, need not be
+included in conveying the object code work.
+
+ A "User Product" is either (1) a "consumer product", which means any
+tangible personal property which is normally used for personal, family,
+or household purposes, or (2) anything designed or sold for incorporation
+into a dwelling. In determining whether a product is a consumer product,
+doubtful cases shall be resolved in favor of coverage. For a particular
+product received by a particular user, "normally used" refers to a
+typical or common use of that class of product, regardless of the status
+of the particular user or of the way in which the particular user
+actually uses, or expects or is expected to use, the product. A product
+is a consumer product regardless of whether the product has substantial
+commercial, industrial or non-consumer uses, unless such uses represent
+the only significant mode of use of the product.
+
+ "Installation Information" for a User Product means any methods,
+procedures, authorization keys, or other information required to install
+and execute modified versions of a covered work in that User Product from
+a modified version of its Corresponding Source. The information must
+suffice to ensure that the continued functioning of the modified object
+code is in no case prevented or interfered with solely because
+modification has been made.
+
+ If you convey an object code work under this section in, or with, or
+specifically for use in, a User Product, and the conveying occurs as
+part of a transaction in which the right of possession and use of the
+User Product is transferred to the recipient in perpetuity or for a
+fixed term (regardless of how the transaction is characterized), the
+Corresponding Source conveyed under this section must be accompanied
+by the Installation Information. But this requirement does not apply
+if neither you nor any third party retains the ability to install
+modified object code on the User Product (for example, the work has
+been installed in ROM).
+
+ The requirement to provide Installation Information does not include a
+requirement to continue to provide support service, warranty, or updates
+for a work that has been modified or installed by the recipient, or for
+the User Product in which it has been modified or installed. Access to a
+network may be denied when the modification itself materially and
+adversely affects the operation of the network or violates the rules and
+protocols for communication across the network.
+
+ Corresponding Source conveyed, and Installation Information provided,
+in accord with this section must be in a format that is publicly
+documented (and with an implementation available to the public in
+source code form), and must require no special password or key for
+unpacking, reading or copying.
+
+ 7. Additional Terms.
+
+ "Additional permissions" are terms that supplement the terms of this
+License by making exceptions from one or more of its conditions.
+Additional permissions that are applicable to the entire Program shall
+be treated as though they were included in this License, to the extent
+that they are valid under applicable law. If additional permissions
+apply only to part of the Program, that part may be used separately
+under those permissions, but the entire Program remains governed by
+this License without regard to the additional permissions.
+
+ When you convey a copy of a covered work, you may at your option
+remove any additional permissions from that copy, or from any part of
+it. (Additional permissions may be written to require their own
+removal in certain cases when you modify the work.) You may place
+additional permissions on material, added by you to a covered work,
+for which you have or can give appropriate copyright permission.
+
+ Notwithstanding any other provision of this License, for material you
+add to a covered work, you may (if authorized by the copyright holders of
+that material) supplement the terms of this License with terms:
+
+ a) Disclaiming warranty or limiting liability differently from the
+ terms of sections 15 and 16 of this License; or
+
+ b) Requiring preservation of specified reasonable legal notices or
+ author attributions in that material or in the Appropriate Legal
+ Notices displayed by works containing it; or
+
+ c) Prohibiting misrepresentation of the origin of that material, or
+ requiring that modified versions of such material be marked in
+ reasonable ways as different from the original version; or
+
+ d) Limiting the use for publicity purposes of names of licensors or
+ authors of the material; or
+
+ e) Declining to grant rights under trademark law for use of some
+ trade names, trademarks, or service marks; or
+
+ f) Requiring indemnification of licensors and authors of that
+ material by anyone who conveys the material (or modified versions of
+ it) with contractual assumptions of liability to the recipient, for
+ any liability that these contractual assumptions directly impose on
+ those licensors and authors.
+
+ All other non-permissive additional terms are considered "further
+restrictions" within the meaning of section 10. If the Program as you
+received it, or any part of it, contains a notice stating that it is
+governed by this License along with a term that is a further
+restriction, you may remove that term. If a license document contains
+a further restriction but permits relicensing or conveying under this
+License, you may add to a covered work material governed by the terms
+of that license document, provided that the further restriction does
+not survive such relicensing or conveying.
+
+ If you add terms to a covered work in accord with this section, you
+must place, in the relevant source files, a statement of the
+additional terms that apply to those files, or a notice indicating
+where to find the applicable terms.
+
+ Additional terms, permissive or non-permissive, may be stated in the
+form of a separately written license, or stated as exceptions;
+the above requirements apply either way.
+
+ 8. Termination.
+
+ You may not propagate or modify a covered work except as expressly
+provided under this License. Any attempt otherwise to propagate or
+modify it is void, and will automatically terminate your rights under
+this License (including any patent licenses granted under the third
+paragraph of section 11).
+
+ However, if you cease all violation of this License, then your
+license from a particular copyright holder is reinstated (a)
+provisionally, unless and until the copyright holder explicitly and
+finally terminates your license, and (b) permanently, if the copyright
+holder fails to notify you of the violation by some reasonable means
+prior to 60 days after the cessation.
+
+ Moreover, your license from a particular copyright holder is
+reinstated permanently if the copyright holder notifies you of the
+violation by some reasonable means, this is the first time you have
+received notice of violation of this License (for any work) from that
+copyright holder, and you cure the violation prior to 30 days after
+your receipt of the notice.
+
+ Termination of your rights under this section does not terminate the
+licenses of parties who have received copies or rights from you under
+this License. If your rights have been terminated and not permanently
+reinstated, you do not qualify to receive new licenses for the same
+material under section 10.
+
+ 9. Acceptance Not Required for Having Copies.
+
+ You are not required to accept this License in order to receive or
+run a copy of the Program. Ancillary propagation of a covered work
+occurring solely as a consequence of using peer-to-peer transmission
+to receive a copy likewise does not require acceptance. However,
+nothing other than this License grants you permission to propagate or
+modify any covered work. These actions infringe copyright if you do
+not accept this License. Therefore, by modifying or propagating a
+covered work, you indicate your acceptance of this License to do so.
+
+ 10. Automatic Licensing of Downstream Recipients.
+
+ Each time you convey a covered work, the recipient automatically
+receives a license from the original licensors, to run, modify and
+propagate that work, subject to this License. You are not responsible
+for enforcing compliance by third parties with this License.
+
+ An "entity transaction" is a transaction transferring control of an
+organization, or substantially all assets of one, or subdividing an
+organization, or merging organizations. If propagation of a covered
+work results from an entity transaction, each party to that
+transaction who receives a copy of the work also receives whatever
+licenses to the work the party's predecessor in interest had or could
+give under the previous paragraph, plus a right to possession of the
+Corresponding Source of the work from the predecessor in interest, if
+the predecessor has it or can get it with reasonable efforts.
+
+ You may not impose any further restrictions on the exercise of the
+rights granted or affirmed under this License. For example, you may
+not impose a license fee, royalty, or other charge for exercise of
+rights granted under this License, and you may not initiate litigation
+(including a cross-claim or counterclaim in a lawsuit) alleging that
+any patent claim is infringed by making, using, selling, offering for
+sale, or importing the Program or any portion of it.
+
+ 11. Patents.
+
+ A "contributor" is a copyright holder who authorizes use under this
+License of the Program or a work on which the Program is based. The
+work thus licensed is called the contributor's "contributor version".
+
+ A contributor's "essential patent claims" are all patent claims
+owned or controlled by the contributor, whether already acquired or
+hereafter acquired, that would be infringed by some manner, permitted
+by this License, of making, using, or selling its contributor version,
+but do not include claims that would be infringed only as a
+consequence of further modification of the contributor version. For
+purposes of this definition, "control" includes the right to grant
+patent sublicenses in a manner consistent with the requirements of
+this License.
+
+ Each contributor grants you a non-exclusive, worldwide, royalty-free
+patent license under the contributor's essential patent claims, to
+make, use, sell, offer for sale, import and otherwise run, modify and
+propagate the contents of its contributor version.
+
+ In the following three paragraphs, a "patent license" is any express
+agreement or commitment, however denominated, not to enforce a patent
+(such as an express permission to practice a patent or covenant not to
+sue for patent infringement). To "grant" such a patent license to a
+party means to make such an agreement or commitment not to enforce a
+patent against the party.
+
+ If you convey a covered work, knowingly relying on a patent license,
+and the Corresponding Source of the work is not available for anyone
+to copy, free of charge and under the terms of this License, through a
+publicly available network server or other readily accessible means,
+then you must either (1) cause the Corresponding Source to be so
+available, or (2) arrange to deprive yourself of the benefit of the
+patent license for this particular work, or (3) arrange, in a manner
+consistent with the requirements of this License, to extend the patent
+license to downstream recipients. "Knowingly relying" means you have
+actual knowledge that, but for the patent license, your conveying the
+covered work in a country, or your recipient's use of the covered work
+in a country, would infringe one or more identifiable patents in that
+country that you have reason to believe are valid.
+
+ If, pursuant to or in connection with a single transaction or
+arrangement, you convey, or propagate by procuring conveyance of, a
+covered work, and grant a patent license to some of the parties
+receiving the covered work authorizing them to use, propagate, modify
+or convey a specific copy of the covered work, then the patent license
+you grant is automatically extended to all recipients of the covered
+work and works based on it.
+
+ A patent license is "discriminatory" if it does not include within
+the scope of its coverage, prohibits the exercise of, or is
+conditioned on the non-exercise of one or more of the rights that are
+specifically granted under this License. You may not convey a covered
+work if you are a party to an arrangement with a third party that is
+in the business of distributing software, under which you make payment
+to the third party based on the extent of your activity of conveying
+the work, and under which the third party grants, to any of the
+parties who would receive the covered work from you, a discriminatory
+patent license (a) in connection with copies of the covered work
+conveyed by you (or copies made from those copies), or (b) primarily
+for and in connection with specific products or compilations that
+contain the covered work, unless you entered into that arrangement,
+or that patent license was granted, prior to 28 March 2007.
+
+ Nothing in this License shall be construed as excluding or limiting
+any implied license or other defenses to infringement that may
+otherwise be available to you under applicable patent law.
+
+ 12. No Surrender of Others' Freedom.
+
+ If conditions are imposed on you (whether by court order, agreement or
+otherwise) that contradict the conditions of this License, they do not
+excuse you from the conditions of this License. If you cannot convey a
+covered work so as to satisfy simultaneously your obligations under this
+License and any other pertinent obligations, then as a consequence you may
+not convey it at all. For example, if you agree to terms that obligate you
+to collect a royalty for further conveying from those to whom you convey
+the Program, the only way you could satisfy both those terms and this
+License would be to refrain entirely from conveying the Program.
+
+ 13. Remote Network Interaction; Use with the GNU General Public License.
+
+ Notwithstanding any other provision of this License, if you modify the
+Program, your modified version must prominently offer all users
+interacting with it remotely through a computer network (if your version
+supports such interaction) an opportunity to receive the Corresponding
+Source of your version by providing access to the Corresponding Source
+from a network server at no charge, through some standard or customary
+means of facilitating copying of software. This Corresponding Source
+shall include the Corresponding Source for any work covered by version 3
+of the GNU General Public License that is incorporated pursuant to the
+following paragraph.
+
+ Notwithstanding any other provision of this License, you have
+permission to link or combine any covered work with a work licensed
+under version 3 of the GNU General Public License into a single
+combined work, and to convey the resulting work. The terms of this
+License will continue to apply to the part which is the covered work,
+but the work with which it is combined will remain governed by version
+3 of the GNU General Public License.
+
+ 14. Revised Versions of this License.
+
+ The Free Software Foundation may publish revised and/or new versions of
+the GNU Affero General Public License from time to time. Such new versions
+will be similar in spirit to the present version, but may differ in detail to
+address new problems or concerns.
+
+ Each version is given a distinguishing version number. If the
+Program specifies that a certain numbered version of the GNU Affero General
+Public License "or any later version" applies to it, you have the
+option of following the terms and conditions either of that numbered
+version or of any later version published by the Free Software
+Foundation. If the Program does not specify a version number of the
+GNU Affero General Public License, you may choose any version ever published
+by the Free Software Foundation.
+
+ If the Program specifies that a proxy can decide which future
+versions of the GNU Affero General Public License can be used, that proxy's
+public statement of acceptance of a version permanently authorizes you
+to choose that version for the Program.
+
+ Later license versions may give you additional or different
+permissions. However, no additional obligations are imposed on any
+author or copyright holder as a result of your choosing to follow a
+later version.
+
+ 15. Disclaimer of Warranty.
+
+ THERE IS NO WARRANTY FOR THE PROGRAM, TO THE EXTENT PERMITTED BY
+APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT
+HOLDERS AND/OR OTHER PARTIES PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY
+OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO,
+THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
+PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE PROGRAM
+IS WITH YOU. SHOULD THE PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF
+ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
+
+ 16. Limitation of Liability.
+
+ IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING
+WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MODIFIES AND/OR CONVEYS
+THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY
+GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE
+USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED TO LOSS OF
+DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD
+PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER PROGRAMS),
+EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF
+SUCH DAMAGES.
+
+ 17. Interpretation of Sections 15 and 16.
+
+ If the disclaimer of warranty and limitation of liability provided
+above cannot be given local legal effect according to their terms,
+reviewing courts shall apply local law that most closely approximates
+an absolute waiver of all civil liability in connection with the
+Program, unless a warranty or assumption of liability accompanies a
+copy of the Program in return for a fee.
+
+ END OF TERMS AND CONDITIONS
+
+ How to Apply These Terms to Your New Programs
+
+ If you develop a new program, and you want it to be of the greatest
+possible use to the public, the best way to achieve this is to make it
+free software which everyone can redistribute and change under these terms.
+
+ To do so, attach the following notices to the program. It is safest
+to attach them to the start of each source file to most effectively
+state the exclusion of warranty; and each file should have at least
+the "copyright" line and a pointer to where the full notice is found.
+
+
+ Copyright (C)
+
+ This program is free software: you can redistribute it and/or modify
+ it under the terms of the GNU Affero General Public License as published
+ by the Free Software Foundation, either version 3 of the License, or
+ (at your option) any later version.
+
+ This program is distributed in the hope that it will be useful,
+ but WITHOUT ANY WARRANTY; without even the implied warranty of
+ MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ GNU Affero General Public License for more details.
+
+ You should have received a copy of the GNU Affero General Public License
+ along with this program. If not, see .
+
+Also add information on how to contact you by electronic and paper mail.
+
+ If your software can interact with users remotely through a computer
+network, you should also make sure that it provides a way for users to
+get its source. For example, if your program is a web application, its
+interface could display a "Source" link that leads users to an archive
+of the code. There are many ways you could offer source, and different
+solutions will be better for different programs; see section 13 for the
+specific requirements.
+
+ You should also get your employer (if you work as a programmer) or school,
+if any, to sign a "copyright disclaimer" for the program, if necessary.
+For more information on this, and how to apply and follow the GNU AGPL, see
+.
diff --git a/libs/sqlite_orm-1.8.2/README.md b/libs/sqlite_orm-1.8.2/README.md
new file mode 100644
index 0000000..9fbe234
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/README.md
@@ -0,0 +1,813 @@
+
+
+
+
+[](https://en.cppreference.com/w/)
+[](https://www.sqlite.org/index.html)
+[](https://github.com/fnc12/sqlite_orm/actions)
+[](https://github.com/fnc12/sqlite_orm/blob/dev/CMakeLists.txt)
+[](https://stackoverflow.com/search?q=sqlite_orm)
+[](https://paypal.me/fnc12)
+[](https://twitter.com/sqlite_orm)
+[](https://patreon.com/fnc12)
+
+# SQLite ORM
+SQLite ORM light header only library for modern C++. Please read the license precisely. The project has AGPL license for open source project and MIT license after purchasing it for 50$ (using [PayPal](https://paypal.me/fnc12) or any different way (contact using email fnc12@me.com)).
+
+# Status
+| Branch | Travis | Appveyor |
+| :----- | :----- | :------- |
+| [`master`](https://github.com/fnc12/sqlite_orm/tree/master) | [](https://travis-ci.org/fnc12/sqlite_orm) | [](https://ci.appveyor.com/project/fnc12/sqlite-orm/history) | | | [](https://github.com/fnc12/sqlite_orm/) |
+| [`dev`](https://github.com/fnc12/sqlite_orm/tree/dev) | [](https://travis-ci.org/fnc12/sqlite_orm) | [](https://ci.appveyor.com/project/fnc12/sqlite-orm/history) | | | [](https://github.com/fnc12/sqlite_orm/tree/dev) |
+
+# Advantages
+
+* **No raw string queries**
+* **Intuitive syntax**
+* **Comfortable interface - one code line per single query**
+* **Built with modern C++14 features (no macros and external scripts)**
+* **CRUD support**
+* **Pure select query support**
+* **Prepared statements support**
+* **UNION, EXCEPT and INTERSECT support**
+* **STL compatible**
+* **Custom types binding support**
+* **BLOB support** - maps to `std::vector` or one can bind your custom type
+* **FOREIGN KEY support**
+* **Composite key support**
+* **JOIN support**
+* **Transactions support**
+* **Migrations functionality**
+* **Powerful conditions**
+* **ORDER BY and LIMIT, OFFSET support**
+* **GROUP BY / DISTINCT support**
+* **INDEX support**
+* **Follows single responsibility principle** - no need write code inside your data model classes
+* **Easy integration** - single header only lib.
+* **The only dependency** - libsqlite3
+* **C++ standard code style**
+* **In memory database support** - provide `:memory:` or empty filename
+* **COLLATE support**
+* **Limits setting/getting support**
+* **User defined functions support**
+
+`sqlite_orm` library allows to create easy data model mappings to your database schema. It is built to manage (CRUD) objects with a primary key and without it. It also allows you to specify table names and column names explicitly no matter how your classes actually named. Take a look at example:
+
+```c++
+
+struct User{
+ int id;
+ std::string firstName;
+ std::string lastName;
+ int birthDate;
+ std::unique_ptr imageUrl;
+ int typeId;
+};
+
+struct UserType {
+ int id;
+ std::string name;
+};
+
+```
+
+So we have database with predefined schema like
+
+`CREATE TABLE users (id integer primary key autoincrement, first_name text not null, last_name text not null, birth_date integer not null, image_url text, type_id integer not null)`
+
+`CREATE TABLE user_types (id integer primary key autoincrement, name text not null DEFAULT 'name_placeholder')`
+
+Now we tell `sqlite_orm` library about our schema and provide database filename. We create `storage` service object that has CRUD interface. Also we create every table and every column. All code is intuitive and minimalistic.
+
+```c++
+
+using namespace sqlite_orm;
+auto storage = make_storage("db.sqlite",
+ make_table("users",
+ make_column("id", &User::id, primary_key().autoincrement()),
+ make_column("first_name", &User::firstName),
+ make_column("last_name", &User::lastName),
+ make_column("birth_date", &User::birthDate),
+ make_column("image_url", &User::imageUrl),
+ make_column("type_id", &User::typeId)),
+ make_table("user_types",
+ make_column("id", &UserType::id, primary_key().autoincrement()),
+ make_column("name", &UserType::name, default_value("name_placeholder"))));
+```
+
+Too easy isn't it? You do not have to specify mapped type explicitly - it is deduced from your member pointers you pass during making a column (for example: `&User::id`). To create a column you have to pass two arguments at least: its name in the table and your mapped class member pointer. You can also add extra arguments to tell your storage about column's constraints like `primary_key`, `autoincrement`, `default_value`, `unique` or `generated_always_as` (order isn't important; `not_null` is deduced from type automatically).
+
+More details about making storage can be found in [tutorial](https://github.com/fnc12/sqlite_orm/wiki/Making-a-storage).
+
+If your datamodel classes have private or protected members to map to sqlite then you can make a storage with setter and getter functions. More info in the [example](https://github.com/fnc12/sqlite_orm/blob/master/examples/private_class_members.cpp).
+
+# CRUD
+
+Let's create and insert new `User` into our database. First we need to create a `User` object with any id and call `insert` function. It will return id of just created user or throw exception if something goes wrong.
+
+```c++
+User user{-1, "Jonh", "Doe", 664416000, std::make_unique("url_to_heaven"), 3 };
+
+auto insertedId = storage.insert(user);
+cout << "insertedId = " << insertedId << endl; // insertedId = 8
+user.id = insertedId;
+
+User secondUser{-1, "Alice", "Inwonder", 831168000, {} , 2};
+insertedId = storage.insert(secondUser);
+secondUser.id = insertedId;
+
+```
+
+Note: if we need to insert a new user with specified id call `storage.replace(user);` instead of `insert`.
+
+Next let's get our user by id.
+
+```c++
+try{
+ auto user = storage.get(insertedId);
+ cout << "user = " << user.firstName << " " << user.lastName << endl;
+}catch(std::system_error e) {
+ cout << e.what() << endl;
+}catch(...){
+ cout << "unknown exeption" << endl;
+}
+```
+
+Probably you may not like throwing exceptions. Me too. Exception `std::system_error` is thrown because return type in `get` function is not nullable. You can use alternative version `get_pointer` which returns `std::unique_ptr` and doesn't throw `not_found_exception` if nothing found - just returns `nullptr`.
+
+```c++
+if(auto user = storage.get_pointer(insertedId)){
+ cout << "user = " << user->firstName << " " << user->lastName << endl;
+}else{
+ cout << "no user with id " << insertedId << endl;
+}
+```
+
+`std::unique_ptr` is used as optional in `sqlite_orm`. Of course there is class optional in C++14 located at `std::experimental::optional`. But we don't want to use it until it is `experimental`.
+
+We can also update our user. It updates row by id provided in `user` object and sets all other non `primary_key` fields to values stored in the passed `user` object. So you can just assign members to `user` object you want and call `update`
+
+```c++
+user.firstName = "Nicholas";
+user.imageUrl = "https://cdn1.iconfinder.com/data/icons/man-icon-set/100/man_icon-21-512.png"
+storage.update(user);
+```
+
+Also there is a non-CRUD update version `update_all`:
+
+```c++
+storage.update_all(set(c(&User::lastName) = "Hardey",
+ c(&User::typeId) = 2),
+ where(c(&User::firstName) == "Tom"));
+```
+
+And delete. To delete you have to pass id only, not whole object. Also we need to explicitly tell which class of object we want to delete. Function name is `remove` not `delete` cause `delete` is a reserved word in C++.
+
+```c++
+storage.remove(insertedId)
+```
+
+Also we can extract all objects into `std::vector`.
+
+```c++
+auto allUsers = storage.get_all();
+cout << "allUsers (" << allUsers.size() << "):" << endl;
+for(auto &user : allUsers) {
+ cout << storage.dump(user) << endl; // dump returns std::string with json-like style object info. For example: { id : '1', first_name : 'Jonh', last_name : 'Doe', birth_date : '664416000', image_url : 'https://cdn1.iconfinder.com/data/icons/man-icon-set/100/man_icon-21-512.png', type_id : '3' }
+}
+```
+
+And one can specify return container type explicitly: let's get all users in `std::list`, not `std::vector`:
+
+```c++
+auto allUsersList = storage.get_all>();
+```
+
+Container must be STL compatible (must have `push_back(T&&)` function in this case).
+
+`get_all` can be too heavy for memory so you can iterate row by row (i.e. object by object):
+
+```c++
+for(auto &user : storage.iterate()) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+`iterate` member function returns adapter object that has `begin` and `end` member functions returning iterators that fetch object on dereference operator call.
+
+CRUD functions `get`, `get_pointer`, `remove`, `update` (not `insert`) work only if your type has a primary key column. If you try to `get` an object that is mapped to your storage but has no primary key column a `std::system_error` will be thrown cause `sqlite_orm` cannot detect an id. If you want to know how to perform a storage without primary key take a look at `date_time.cpp` example in `examples` folder.
+
+# Prepared statements
+
+Prepared statements are strongly typed.
+
+```c++
+// SELECT doctor_id
+// FROM visits
+// WHERE LENGTH(patient_name) > 8
+auto selectStatement = storage.prepare(select(&Visit::doctor_id, where(length(&Visit::patient_name) > 8)));
+cout << "selectStatement = " << selectStatement.sql() << endl; // prints "SELECT doctor_id FROM ..."
+auto rows = storage.execute(selectStatement); // rows is std::vector
+
+// SELECT doctor_id
+// FROM visits
+// WHERE LENGTH(patient_name) > 11
+get<0>(selectStatement) = 11;
+auto rows2 = storage.execute(selectStatement);
+```
+`get(statement)` function call allows you to access fields to bind them to your statement.
+
+# Aggregate Functions
+
+```c++
+// SELECT AVG(id) FROM users
+auto averageId = storage.avg(&User::id);
+cout << "averageId = " << averageId << endl; // averageId = 4.5
+
+// SELECT AVG(birth_date) FROM users
+auto averageBirthDate = storage.avg(&User::birthDate);
+cout << "averageBirthDate = " << averageBirthDate << endl; // averageBirthDate = 6.64416e+08
+
+// SELECT COUNT(*) FROM users
+auto usersCount = storage.count();
+cout << "users count = " << usersCount << endl; // users count = 8
+
+// SELECT COUNT(id) FROM users
+auto countId = storage.count(&User::id);
+cout << "countId = " << countId << endl; // countId = 8
+
+// SELECT COUNT(image_url) FROM users
+auto countImageUrl = storage.count(&User::imageUrl);
+cout << "countImageUrl = " << countImageUrl << endl; // countImageUrl = 5
+
+// SELECT GROUP_CONCAT(id) FROM users
+auto concatedUserId = storage.group_concat(&User::id);
+cout << "concatedUserId = " << concatedUserId << endl; // concatedUserId = 1,2,3,4,5,6,7,8
+
+// SELECT GROUP_CONCAT(id, "---") FROM users
+auto concatedUserIdWithDashes = storage.group_concat(&User::id, "---");
+cout << "concatedUserIdWithDashes = " << concatedUserIdWithDashes << endl; // concatedUserIdWithDashes = 1---2---3---4---5---6---7---8
+
+// SELECT MAX(id) FROM users
+if(auto maxId = storage.max(&User::id)){
+ cout << "maxId = " << *maxId <)
+}else{
+ cout << "maxId is null" << endl;
+}
+
+// SELECT MAX(first_name) FROM users
+if(auto maxFirstName = storage.max(&User::firstName)){
+ cout << "maxFirstName = " << *maxFirstName << endl; // maxFirstName = Jonh (maxFirstName is std::unique_ptr)
+}else{
+ cout << "maxFirstName is null" << endl;
+}
+
+// SELECT MIN(id) FROM users
+if(auto minId = storage.min(&User::id)){
+ cout << "minId = " << *minId << endl; // minId = 1 (minId is std::unique_ptr)
+}else{
+ cout << "minId is null" << endl;
+}
+
+// SELECT MIN(last_name) FROM users
+if(auto minLastName = storage.min(&User::lastName)){
+ cout << "minLastName = " << *minLastName << endl; // minLastName = Doe
+}else{
+ cout << "minLastName is null" << endl;
+}
+
+// SELECT SUM(id) FROM users
+if(auto sumId = storage.sum(&User::id)){ // sumId is std::unique_ptr
+ cout << "sumId = " << *sumId << endl;
+}else{
+ cout << "sumId is null" << endl;
+}
+
+// SELECT TOTAL(id) FROM users
+auto totalId = storage.total(&User::id);
+cout << "totalId = " << totalId << endl; // totalId is double (always)
+```
+
+# Where conditions
+
+You also can select objects with custom where conditions with `=`, `!=`, `>`, `>=`, `<`, `<=`, `IN`, `BETWEEN` and `LIKE`.
+
+For example: let's select users with id lesser than 10:
+
+```c++
+// SELECT * FROM users WHERE id < 10
+auto idLesserThan10 = storage.get_all(where(c(&User::id) < 10));
+cout << "idLesserThan10 count = " << idLesserThan10.size() << endl;
+for(auto &user : idLesserThan10) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+Or select all users who's first name is not equal "John":
+
+```c++
+// SELECT * FROM users WHERE first_name != 'John'
+auto notJohn = storage.get_all(where(c(&User::firstName) != "John"));
+cout << "notJohn count = " << notJohn.size() << endl;
+for(auto &user : notJohn) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+By the way one can implement not equal in a different way using C++ negation operator:
+
+```c++
+auto notJohn2 = storage.get_all(where(not (c(&User::firstName) == "John")));
+```
+
+You can use `!` and `not` in this case cause they are equal. Also you can chain several conditions with `and` and `or` operators. Let's try to get users with query with conditions like `where id >= 5 and id <= 7 and not id = 6`:
+
+```c++
+auto id5and7 = storage.get_all(where(c(&User::id) <= 7 and c(&User::id) >= 5 and not (c(&User::id) == 6)));
+cout << "id5and7 count = " << id5and7.size() << endl;
+for(auto &user : id5and7) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+Or let's just export two users with id 10 or id 16 (of course if these users exist):
+
+```c++
+auto id10or16 = storage.get_all(where(c(&User::id) == 10 or c(&User::id) == 16));
+cout << "id10or16 count = " << id10or16.size() << endl;
+for(auto &user : id10or16) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+In fact you can chain together any number of different conditions with any operator from `and`, `or` and `not`. All conditions are templated so there is no runtime overhead. And this makes `sqlite_orm` the most powerful **sqlite** C++ ORM library!
+
+Moreover you can use parentheses to set the priority of query conditions:
+
+```c++
+auto cuteConditions = storage.get_all(where((c(&User::firstName) == "John" or c(&User::firstName) == "Alex") and c(&User::id) == 4)); // where (first_name = 'John' or first_name = 'Alex') and id = 4
+cout << "cuteConditions count = " << cuteConditions.size() << endl; // cuteConditions count = 1
+cuteConditions = storage.get_all(where(c(&User::firstName) == "John" or (c(&User::firstName) == "Alex" and c(&User::id) == 4))); // where first_name = 'John' or (first_name = 'Alex' and id = 4)
+cout << "cuteConditions count = " << cuteConditions.size() << endl; // cuteConditions count = 2
+```
+
+Also we can implement `get` by id with `get_all` and `where` like this:
+
+```c++
+// SELECT * FROM users WHERE ( 2 = id )
+auto idEquals2 = storage.get_all(where(2 == c(&User::id)));
+cout << "idEquals2 count = " << idEquals2.size() << endl;
+if(idEquals2.size()){
+ cout << storage.dump(idEquals2.front()) << endl;
+}else{
+ cout << "user with id 2 doesn't exist" << endl;
+}
+```
+
+Lets try the `IN` operator:
+
+```c++
+// SELECT * FROM users WHERE id IN (2, 4, 6, 8, 10)
+auto evenLesserTen10 = storage.get_all(where(in(&User::id, {2, 4, 6, 8, 10})));
+cout << "evenLesserTen10 count = " << evenLesserTen10.size() << endl;
+for(auto &user : evenLesserTen10) {
+ cout << storage.dump(user) << endl;
+}
+
+// SELECT * FROM users WHERE last_name IN ("Doe", "White")
+auto doesAndWhites = storage.get_all(where(in(&User::lastName, {"Doe", "White"})));
+cout << "doesAndWhites count = " << doesAndWhites.size() << endl;
+for(auto &user : doesAndWhites) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+And `BETWEEN`:
+
+```c++
+// SELECT * FROM users WHERE id BETWEEN 66 AND 68
+auto betweenId = storage.get_all(where(between(&User::id, 66, 68)));
+cout << "betweenId = " << betweenId.size() << endl;
+for(auto &user : betweenId) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+And even `LIKE`:
+
+```c++
+// SELECT * FROM users WHERE last_name LIKE 'D%'
+auto whereNameLike = storage.get_all(where(like(&User::lastName, "D%")));
+cout << "whereNameLike = " << whereNameLike.size() << endl;
+for(auto &user : whereNameLike) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+Looks like magic but it works very simple. Cute function `c` (column) takes a class member pointer and returns a special expression middle object that can be used with operators overloaded in `::sqlite_orm` namespace. Operator overloads act just like functions
+
+* is_equal
+* is_not_equal
+* greater_than
+* greater_or_equal
+* lesser_than
+* lesser_or_equal
+* is_null
+* is_not_null
+
+that simulate binary comparison operator so they take 2 arguments: left hand side and right hand side. Arguments may be either member pointer of mapped class or any other expression (core/aggregate function, literal or subexpression). Binary comparison functions map arguments to text to be passed to sqlite engine to process query. Member pointers are being mapped to column names and literals/variables/constants to '?' and then are bound automatically. Next `where` function places brackets around condition and adds "WHERE" keyword before condition text. Next resulted string appends to a query string and is being processed further.
+
+If you omit `where` function in `get_all` it will return all objects from a table:
+
+```c++
+auto allUsers = storage.get_all();
+```
+
+Also you can use `remove_all` function to perform `DELETE FROM ... WHERE` query with the same type of conditions.
+
+```c++
+storage.remove_all(where(c(&User::id) < 100));
+```
+
+# Raw select
+
+If you need to extract only a single column (`SELECT %column_name% FROM %table_name% WHERE %conditions%`) you can use a non-CRUD `select` function:
+
+```c++
+
+// SELECT id FROM users
+auto allIds = storage.select(&User::id);
+cout << "allIds count = " << allIds.size() << endl; // allIds is std::vector
+for(auto &id : allIds) {
+ cout << id << " ";
+}
+cout << endl;
+
+// SELECT id FROM users WHERE last_name = 'Doe'
+auto doeIds = storage.select(&User::id, where(c(&User::lastName) == "Doe"));
+cout << "doeIds count = " << doeIds.size() << endl; // doeIds is std::vector
+for(auto &doeId : doeIds) {
+ cout << doeId << " ";
+}
+cout << endl;
+
+// SELECT last_name FROM users WHERE id < 300
+auto allLastNames = storage.select(&User::lastName, where(c(&User::id) < 300));
+cout << "allLastNames count = " << allLastNames.size() << endl; // allLastNames is std::vector
+for(auto &lastName : allLastNames) {
+ cout << lastName << " ";
+}
+cout << endl;
+
+// SELECT id FROM users WHERE image_url IS NULL
+auto idsWithoutUrls = storage.select(&User::id, where(is_null(&User::imageUrl)));
+for(auto id : idsWithoutUrls) {
+ cout << "id without image url " << id << endl;
+}
+
+// SELECT id FROM users WHERE image_url IS NOT NULL
+auto idsWithUrl = storage.select(&User::id, where(is_not_null(&User::imageUrl)));
+for(auto id : idsWithUrl) {
+ cout << "id with image url " << id << endl;
+}
+auto idsWithUrl2 = storage.select(&User::id, where(not is_null(&User::imageUrl)));
+assert(std::equal(idsWithUrl2.begin(),
+ idsWithUrl2.end(),
+ idsWithUrl.begin()));
+```
+
+Also you're able to select several column in a vector of tuples. Example:
+
+```c++
+// `SELECT first_name, last_name FROM users WHERE id > 250 ORDER BY id`
+auto partialSelect = storage.select(columns(&User::firstName, &User::lastName),
+ where(c(&User::id) > 250),
+ order_by(&User::id));
+cout << "partialSelect count = " << partialSelect.size() << endl;
+for(auto &t : partialSelect) {
+ auto &firstName = std::get<0>(t);
+ auto &lastName = std::get<1>(t);
+ cout << firstName << " " << lastName << endl;
+}
+```
+
+# ORDER BY support
+
+ORDER BY query option can be applied to `get_all` and `select` functions just like `where` but with `order_by` function. It can be mixed with WHERE in a single query. Examples:
+
+```c++
+// `SELECT * FROM users ORDER BY id`
+auto orderedUsers = storage.get_all(order_by(&User::id));
+cout << "orderedUsers count = " << orderedUsers.size() << endl;
+for(auto &user : orderedUsers) {
+ cout << storage.dump(user) << endl;
+}
+
+// `SELECT * FROM users WHERE id < 250 ORDER BY first_name`
+auto orderedUsers2 = storage.get_all(where(c(&User::id) < 250), order_by(&User::firstName));
+cout << "orderedUsers2 count = " << orderedUsers2.size() << endl;
+for(auto &user : orderedUsers2) {
+ cout << storage.dump(user) << endl;
+}
+
+// `SELECT * FROM users WHERE id > 100 ORDER BY first_name ASC`
+auto orderedUsers3 = storage.get_all(where(c(&User::id) > 100), order_by(&User::firstName).asc());
+cout << "orderedUsers3 count = " << orderedUsers3.size() << endl;
+for(auto &user : orderedUsers3) {
+ cout << storage.dump(user) << endl;
+}
+
+// `SELECT * FROM users ORDER BY id DESC`
+auto orderedUsers4 = storage.get_all(order_by(&User::id).desc());
+cout << "orderedUsers4 count = " << orderedUsers4.size() << endl;
+for(auto &user : orderedUsers4) {
+ cout << storage.dump(user) << endl;
+}
+
+// `SELECT first_name FROM users ORDER BY ID DESC`
+auto orderedFirstNames = storage.select(&User::firstName, order_by(&User::id).desc());
+cout << "orderedFirstNames count = " << orderedFirstNames.size() << endl;
+for(auto &firstName : orderedFirstNames) {
+ cout << "firstName = " << firstName << endl;
+}
+```
+
+# LIMIT and OFFSET
+
+There are three available versions of `LIMIT`/`OFFSET` options:
+
+- LIMIT %limit%
+- LIMIT %limit% OFFSET %offset%
+- LIMIT %offset%, %limit%
+
+All these versions available with the same interface:
+
+```c++
+// `SELECT * FROM users WHERE id > 250 ORDER BY id LIMIT 5`
+auto limited5 = storage.get_all(where(c(&User::id) > 250),
+ order_by(&User::id),
+ limit(5));
+cout << "limited5 count = " << limited5.size() << endl;
+for(auto &user : limited5) {
+ cout << storage.dump(user) << endl;
+}
+
+// `SELECT * FROM users WHERE id > 250 ORDER BY id LIMIT 5, 10`
+auto limited5comma10 = storage.get_all(where(c(&User::id) > 250),
+ order_by(&User::id),
+ limit(5, 10));
+cout << "limited5comma10 count = " << limited5comma10.size() << endl;
+for(auto &user : limited5comma10) {
+ cout << storage.dump(user) << endl;
+}
+
+// `SELECT * FROM users WHERE id > 250 ORDER BY id LIMIT 5 OFFSET 10`
+auto limit5offset10 = storage.get_all(where(c(&User::id) > 250),
+ order_by(&User::id),
+ limit(5, offset(10)));
+cout << "limit5offset10 count = " << limit5offset10.size() << endl;
+for(auto &user : limit5offset10) {
+ cout << storage.dump(user) << endl;
+}
+```
+
+Please beware that queries `LIMIT 5, 10` and `LIMIT 5 OFFSET 10` mean different. `LIMIT 5, 10` means `LIMIT 10 OFFSET 5`.
+
+# JOIN support
+
+You can perform simple `JOIN`, `CROSS JOIN`, `INNER JOIN`, `LEFT JOIN` or `LEFT OUTER JOIN` in your query. Instead of joined table specify mapped type. Example for doctors and visits:
+
+```c++
+// SELECT a.doctor_id, a.doctor_name,
+// c.patient_name, c.vdate
+// FROM doctors a
+// LEFT JOIN visits c
+// ON a.doctor_id=c.doctor_id;
+auto rows = storage2.select(columns(&Doctor::id, &Doctor::name, &Visit::patientName, &Visit::vdate),
+ left_join(on(c(&Doctor::id) == &Visit::doctorId))); // one `c` call is enough cause operator overloads are templated
+for(auto &row : rows) {
+ cout << std::get<0>(row) << '\t' << std::get<1>(row) << '\t' << std::get<2>(row) << '\t' << std::get<3>(row) << endl;
+}
+cout << endl;
+```
+
+Simple `JOIN`:
+
+```c++
+// SELECT a.doctor_id,a.doctor_name,
+// c.patient_name,c.vdate
+// FROM doctors a
+// JOIN visits c
+// ON a.doctor_id=c.doctor_id;
+rows = storage2.select(columns(&Doctor::id, &Doctor::name, &Visit::patientName, &Visit::vdate),
+ join(on(c(&Doctor::id) == &Visit::doctorId)));
+for(auto &row : rows) {
+ cout << std::get<0>(row) << '\t' << std::get<1>(row) << '\t' << std::get<2>(row) << '\t' << std::get<3>(row) << endl;
+}
+cout << endl;
+```
+
+Two `INNER JOIN`s in one query:
+
+```c++
+// SELECT
+// trackid,
+// tracks.name AS Track,
+// albums.title AS Album,
+// artists.name AS Artist
+// FROM
+// tracks
+// INNER JOIN albums ON albums.albumid = tracks.albumid
+// INNER JOIN artists ON artists.artistid = albums.artistid;
+auto innerJoinRows2 = storage.select(columns(&Track::trackId, &Track::name, &Album::title, &Artist::name),
+ inner_join(on(c(&Album::albumId) == &Track::albumId)),
+ inner_join(on(c(&Artist::artistId) == &Album::artistId)));
+// innerJoinRows2 is std::vector>
+```
+
+More join examples can be found in [examples folder](https://github.com/fnc12/sqlite_orm/blob/master/examples/left_and_inner_join.cpp).
+
+# Migrations functionality
+
+There are no explicit `up` and `down` functions that are used to be used in migrations. Instead `sqlite_orm` offers `sync_schema` function that takes responsibility of comparing actual db file schema with one you specified in `make_storage` call and if something is not equal it alters or drops/creates schema.
+
+```c++
+storage.sync_schema();
+// or
+storage.sync_schema(true);
+```
+
+Please beware that `sync_schema` doesn't guarantee that data will be saved. It *tries* to save it only. Below you can see rules list that `sync_schema` follows during call:
+* if there are excess tables exist in db they are ignored (not dropped)
+* every table from storage is compared with it's db analog and
+ * if table doesn't exist it is created
+ * if table exists its colums are being compared with table_info from db and
+ * if there are columns in db that do not exist in storage (excess) table will be dropped and recreated if `preserve` is `false`, and table will be copied into temporary table without excess columns, source table will be dropped, copied table will be renamed to source table (sqlite remove column technique) if `preserve` is `true`. `preserve` is the first argument in `sync_schema` function. It's default value is `false`. Beware that setting it to `true` may take time for copying table rows.
+ * if there are columns in storage that do not exist in db they will be added using 'ALTER TABLE ... ADD COLUMN ...' command and table data will not be dropped but if any of added columns is null but has not default value table will be dropped and recreated
+ * if there is any column existing in both db and storage but differs by any of properties (type, pk, notnull) table will be dropped and recreated (dflt_value isn't checked cause there can be ambiguity in default values, please beware).
+
+The best practice is to call this function right after storage creation.
+
+# Transactions
+
+There are three ways to begin and commit/rollback transactions:
+* explicitly call `begin_transaction();`, `rollback();` or `commit();` functions
+* use `transaction` function which begins transaction implicitly and takes a lambda argument which returns true for commit and false for rollback. All storage calls performed in lambda can be commited or rollbacked by returning `true` or `false`.
+* use `transaction_guard` function which returns a guard object which works just like `lock_guard` for `std::mutex`.
+
+Example for explicit call:
+
+```c++
+auto secondUser = storage.get(2);
+
+storage.begin_transaction();
+secondUser.typeId = 3;
+storage.update(secondUser);
+storage.rollback(); // or storage.commit();
+
+secondUser = storage.get(secondUser.id);
+assert(secondUser.typeId != 3);
+```
+
+Example for implicit call:
+
+```c++
+storage.transaction([&] () mutable { // mutable keyword allows make non-const function calls
+ auto secondUser = storage.get(2);
+ secondUser.typeId = 1;
+ storage.update(secondUser);
+ auto gottaRollback = bool(rand() % 2);
+ if(gottaRollback){ // dummy condition for test
+ return false; // exits lambda and calls ROLLBACK
+ }
+ return true; // exits lambda and calls COMMIT
+});
+```
+
+The second way guarantees that `commit` or `rollback` will be called. You can use either way.
+
+Trancations are useful with `changes` sqlite function that returns number of rows modified.
+
+```c++
+storage.transaction([&] () mutable {
+ storage.remove_all(where(c(&User::id) < 100));
+ auto usersRemoved = storage.changes();
+ cout << "usersRemoved = " << usersRemoved << endl;
+ return true;
+});
+```
+
+It will print a number of deleted users (rows). But if you call `changes` without a transaction and your database is located in file not in RAM the result will be 0 always cause `sqlite_orm` opens and closes connection every time you call a function without a transaction.
+
+Also a `transaction` function returns `true` if transaction is commited and `false` if it is rollbacked. It can be useful if your next actions depend on transaction result:
+
+```c++
+auto commited = storage.transaction([&] () mutable {
+ auto secondUser = storage.get(2);
+ secondUser.typeId = 1;
+ storage.update(secondUser);
+ auto gottaRollback = bool(rand() % 2);
+ if(gottaRollback){ // dummy condition for test
+ return false; // exits lambda and calls ROLLBACK
+ }
+ return true; // exits lambda and calls COMMIT
+});
+if(commited){
+ cout << "Commited successfully, go on." << endl;
+}else{
+ cerr << "Commit failed, process an error" << endl;
+}
+```
+
+Example for `transaction_guard` function:
+
+```c++
+try{
+ auto guard = storage.transaction_guard(); // calls BEGIN TRANSACTION and returns guard object
+ user.name = "Paul";
+ auto notExisting = storage.get(-1); // exception is thrown here, guard calls ROLLBACK in its destructor
+ guard.commit();
+}catch(...){
+ cerr << "exception" << endl;
+}
+```
+
+# In memory database
+
+To manage in memory database just provide `:memory:` or `""` instead as filename to `make_storage`.
+
+# Comparison with other C++ libs
+
+| |sqlite_orm|[SQLiteCpp](https://github.com/SRombauts/SQLiteCpp)|[hiberlite](https://github.com/paulftw/hiberlite)|[ODB](https://www.codesynthesis.com/products/odb/)|
+|---|:---:|:---:|:---:|:---:|
+|Schema sync|yes|no|yes|no|
+|Single responsibility principle|yes|yes|no|no|
+|STL compatible|yes|no|no|no|
+|No raw string queries|yes|no|yes|yes|
+|Transactions|yes|yes|no|yes|
+|Custom types binding|yes|no|yes|yes|
+|Doesn't use macros and/or external codegen scripts|yes|yes|no|no|
+|Aggregate functions|yes|yes|no|yes|
+|Prepared statements|yes|yes|no|no|
+
+# Notes
+
+To work well your data model class must be default constructable and must not have const fields mapped to database cause they are assigned during queries. Otherwise code won't compile on line with member assignment operator.
+
+For more details please check the project [wiki](https://github.com/fnc12/sqlite_orm/wiki).
+
+# Installation
+
+**Note**: Installation is not necessary if you plan to use the fetchContent method, see below in Usage.
+
+Use a popular package manager like [vcpkg](https://github.com/Microsoft/vcpkg) and just install it with the `vcpkg install sqlite-orm` command.
+
+Or you build it from source:
+
+```bash
+git clone https://github.com/fnc12/sqlite_orm.git sqlite_orm
+cd sqlite_orm
+cmake -B build
+cmake --build build --target install
+```
+You might need admin rights for the last command.
+
+# Usage
+
+## CMake
+
+If you use cmake, there are two supported ways how to use it with cmake (if another works as well or should be supported, open an issue).
+
+Either way you choose, the include path as well as the dependency sqlite3 will be set automatically on your target. So usage is straight forward, but you need to have installed sqlite3 on your system (see Requirements below)
+
+## Find Package
+
+If you have installed the lib system wide and it's in your PATH, you can use find_package to include it in cmake. It will make a target `sqlite_orm::sqlite_orm` available which you can link against. Have a look at examples/find_package for a full example.
+
+```cmake
+find_package(SqliteOrm REQUIRED)
+
+target_link_libraries(main PRIVATE sqlite_orm::sqlite_orm)
+```
+
+## Fetch Content (Recommended)
+
+Alternatively, cmake can download the project directly from github during configure stage and therefore you don't need to install the lib before.
+Againt a target `sqlite_orm::sqlite_orm` will be available which you can link against. Have a look at examples/fetch_content for a full example.
+
+## No CMake
+
+If you want to use the lib directly with Make or something else, just set the inlcude path correctly (should be correct on Linux already), so `sqlite_orm/sqlite_orm.h` is found. As this is a header only lib, there is nothing more you have to do.
+
+# Requirements
+
+* C++14 compatible compiler (not C++11 cause of templated lambdas in the lib).
+* Sqlite3 installed on your system and in the path, so cmake can find it (or linked to you project if you don't use cmake)
+
+# Video from conference
+
+[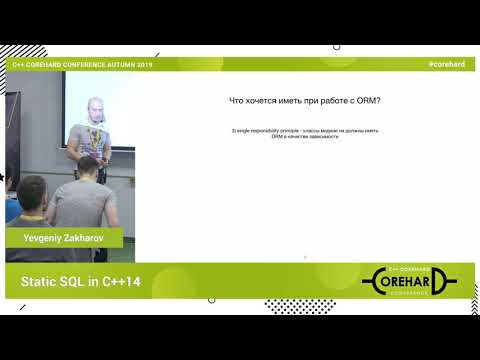](https://www.youtube.com/watch?v=ngsilquWgpo)
+
+# SqliteMan
+
+In case you need a native SQLite client for macOS or Windows 10 you can use SqliteMan https://sqliteman.dev. It is not a commercial. It is a free native client being developed by the maintainer of this repo.
diff --git a/libs/sqlite_orm-1.8.2/TODO.md b/libs/sqlite_orm-1.8.2/TODO.md
new file mode 100644
index 0000000..197a2ca
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/TODO.md
@@ -0,0 +1,27 @@
+# To do list
+
+`sqlite_orm` is a wonderful library but there are still features that are not implemented. Here you can find a list of them:
+
+* `FOREIGN KEY` - sync_schema fk comparison and ability of two tables to have fk to each other (`PRAGMA foreign_key_list(%table_name%);` may be useful)
+* rest of core functions(https://sqlite.org/lang_corefunc.html)
+* `ATTACH`
+* blob incremental I/O https://sqlite.org/c3ref/blob_open.html
+* CREATE VIEW and other view operations https://sqlite.org/lang_createview.html
+* query static check for correct order (e.g. `GROUP BY` after `WHERE`)
+* `WINDOW`
+* `SAVEPOINT` https://www.sqlite.org/lang_savepoint.html
+* add `static_assert` in crud `get*` functions in case user passes `where_t` instead of id to make compilation error more clear (example https://github.com/fnc12/sqlite_orm/issues/485)
+* named constraints: constraint can have name `CREATE TABLE heroes(id INTEGER CONSTRAINT pk PRIMARY KEY)`
+* `FILTER` clause https://sqlite.org/lang_aggfunc.html#aggfilter
+* scalar math functions https://sqlite.org/lang_mathfunc.html
+* improve DROP COLUMN in `sync_schema` https://sqlite.org/lang_altertable.html#altertabdropcol
+* `UPDATE FROM` support https://sqlite.org/lang_update.html#upfrom
+* `iif()` function https://sqlite.org/lang_corefunc.html#iif
+* add strong typed collate syntax (more info [here](https://github.com/fnc12/sqlite_orm/issues/767#issuecomment-887689672))
+* strict tables https://sqlite.org/stricttables.html
+* static assert when UPDATE is called with no PKs
+* `json_each` and `json_tree` functions for JSON1 extension
+* update hook
+* `RAISE`
+
+Please feel free to add any feature that isn't listed here and not implemented yet.
diff --git a/libs/sqlite_orm-1.8.2/_config.yml b/libs/sqlite_orm-1.8.2/_config.yml
new file mode 100644
index 0000000..2f7efbe
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/_config.yml
@@ -0,0 +1 @@
+theme: jekyll-theme-minimal
\ No newline at end of file
diff --git a/libs/sqlite_orm-1.8.2/appveyor.yml b/libs/sqlite_orm-1.8.2/appveyor.yml
new file mode 100644
index 0000000..55bed40
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/appveyor.yml
@@ -0,0 +1,184 @@
+# build format
+version: "{build}"
+
+skip_branch_with_pr: true
+skip_commits:
+ files:
+ - .git*
+ - .travis.yml
+ - _config.yml
+ - LICENSE
+ - '*.md'
+ - '*.png'
+ - '*.sh'
+
+# configurations to add to build matrix
+configuration:
+ #- Debug
+ - Release
+
+environment:
+ appveyor_yml_disable_ps_linux: true
+ matrix:
+ - job_name: clang, C++14
+ appveyor_build_worker_image: Ubuntu
+ CC: clang
+ CXX: clang++
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_14=ON"
+ cmake_build_parallel: --parallel
+
+ - job_name: gcc, C++14
+ appveyor_build_worker_image: Ubuntu
+ CC: gcc
+ CXX: g++
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_14=ON"
+ # gcc was stuck with a parallel build
+ cmake_build_parallel: ""
+
+ # Representative for C++14
+ - job_name: Visual Studio 2017, x64, C++14
+ appveyor_build_worker_image: Visual Studio 2017
+ platform: x64
+ SQLITE_ORM_CXX_STANDARD: ""
+
+ - job_name: clang, C++17
+ appveyor_build_worker_image: Ubuntu
+ CC: clang
+ CXX: clang++
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_17=ON"
+ cmake_build_parallel: --parallel
+
+ - job_name: gcc, C++17
+ appveyor_build_worker_image: Ubuntu
+ CC: gcc
+ CXX: g++
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_17=ON"
+ cmake_build_parallel: ""
+
+ - job_name: clang, C++20 (with examples)
+ appveyor_build_worker_image: Ubuntu
+ CC: clang
+ CXX: clang++
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_20=ON"
+ # clang was stuck with a parallel build of examples
+ cmake_build_parallel: ""
+ cmake_build_examples: "-DBUILD_EXAMPLES=ON"
+
+ - job_name: gcc, C++20
+ appveyor_build_worker_image: Ubuntu
+ CC: gcc
+ CXX: g++
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_20=ON"
+ cmake_build_parallel: ""
+
+ - job_name: Visual Studio 2022, x64, C++17
+ appveyor_build_worker_image: Visual Studio 2022
+ platform: x64
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_17=ON"
+
+ - job_name: Visual Studio 2022, x64, C++20
+ appveyor_build_worker_image: Visual Studio 2022
+ platform: x64
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_20=ON"
+
+ - job_name: Visual Studio 2022, x86, C++20
+ appveyor_build_worker_image: Visual Studio 2022
+ platform: x86
+ SQLITE_ORM_CXX_STANDARD: "-DSQLITE_ORM_ENABLE_CXX_20=ON"
+
+matrix:
+ fast_finish: true
+
+for:
+-
+ # Windows
+ matrix:
+ only:
+ - appveyor_build_worker_image: Visual Studio 2017
+ - appveyor_build_worker_image: Visual Studio 2022
+ init:
+ - |-
+ echo %appveyor_build_worker_image% - %platform% - %configuration%
+ cmake --version
+ if "%platform%"=="x64" (set architecture=-A x64)
+ if "%platform%"=="x86" (set architecture=-A Win32)
+ if "%appveyor_build_worker_image%"=="Visual Studio 2022" (set generator="Visual Studio 17 2022" %architecture%)
+ if "%appveyor_build_worker_image%"=="Visual Studio 2017" (set generator="Visual Studio 15 2017" %architecture%)
+ install:
+ - |-
+ cd C:\Tools\vcpkg
+ git fetch --tags && git checkout 2023.01.09
+ cd %APPVEYOR_BUILD_FOLDER%
+ C:\Tools\vcpkg\bootstrap-vcpkg.bat -disableMetrics
+ C:\Tools\vcpkg\vcpkg integrate install
+ set VCPKG_DEFAULT_TRIPLET=%platform%-windows
+ vcpkg install sqlite3
+ rem The Visual Studio 2017 build worker image comes with CMake 3.16 only, and sqlite_orm will build the Catch2 dependency from source
+ if not "%appveyor_build_worker_image%"=="Visual Studio 2017" (vcpkg install catch2)
+ before_build:
+ - |-
+ mkdir compile
+ cd compile
+ cmake %SQLITE_ORM_CXX_STANDARD% -G %generator% -DCMAKE_TOOLCHAIN_FILE=C:/Tools/vcpkg/scripts/buildsystems/vcpkg.cmake ..
+ # build examples, and run tests (ie make & make test)
+ build_script:
+ - |-
+ cmake --build . --config %configuration% -- /m
+ ctest --verbose --output-on-failure --build-config %configuration%
+
+-
+ # Linux
+ matrix:
+ only:
+ - appveyor_build_worker_image: Ubuntu
+ init:
+ - |-
+ echo $appveyor_build_worker_image
+ $CXX --version
+ cmake --version
+ # using custom vcpkg triplets for building and linking dynamic dependent libraries
+ install:
+ - |-
+ pushd $HOME/vcpkg
+ git fetch --tags && git checkout 2023.01.09
+ popd
+ $HOME/vcpkg/bootstrap-vcpkg.sh -disableMetrics
+ $HOME/vcpkg/vcpkg integrate install --overlay-triplets=vcpkg/triplets
+ vcpkg install sqlite3 catch2 --overlay-triplets=vcpkg/triplets
+ before_build:
+ - |-
+ mkdir compile
+ cd compile
+ cmake $SQLITE_ORM_CXX_STANDARD $cmake_build_examples --toolchain $HOME/vcpkg/scripts/buildsystems/vcpkg.cmake ..
+ # build examples, and run tests (ie make & make test)
+ build_script:
+ - |-
+ cmake --build . $cmake_build_parallel
+ ctest --verbose --output-on-failure
+-
+ # macOS
+ matrix:
+ only:
+ - appveyor_build_worker_image: macOS
+ init:
+ - |-
+ echo $appveyor_build_worker_image
+ $CXX --version
+ cmake --version
+ # using custom vcpkg triplets for building and linking dynamic dependent libraries
+ install:
+ - |-
+ git clone --depth 1 --branch 2023.01.09 https://github.com/microsoft/vcpkg.git $HOME/vcpkg
+ $HOME/vcpkg/bootstrap-vcpkg.sh -disableMetrics
+ $HOME/vcpkg/vcpkg integrate install --overlay-triplets=vcpkg/triplets
+ vcpkg install sqlite3 catch2 --overlay-triplets=vcpkg/triplets
+ before_build:
+ - |-
+ mkdir compile
+ cd compile
+ cmake $SQLITE_ORM_CXX_STANDARD --toolchain $HOME/vcpkg/scripts/buildsystems/vcpkg.cmake ..
+ # build examples, and run tests (ie make & make test)
+ build_script:
+ - |-
+ cmake --build . --parallel
+ ctest --verbose --output-on-failure
diff --git a/libs/sqlite_orm-1.8.2/cmake/SqliteOrmConfig.cmake.in b/libs/sqlite_orm-1.8.2/cmake/SqliteOrmConfig.cmake.in
new file mode 100644
index 0000000..e0635d2
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/cmake/SqliteOrmConfig.cmake.in
@@ -0,0 +1,4 @@
+include(CMakeFindDependencyMacro)
+find_dependency(SQLite3)
+
+include(${CMAKE_CURRENT_LIST_DIR}/SqliteOrmTargets.cmake)
diff --git a/libs/sqlite_orm-1.8.2/cmake/ucm.cmake b/libs/sqlite_orm-1.8.2/cmake/ucm.cmake
new file mode 100755
index 0000000..4947070
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/cmake/ucm.cmake
@@ -0,0 +1,663 @@
+#
+# ucm.cmake - useful cmake macros
+#
+# Copyright (c) 2016 Viktor Kirilov
+#
+# Distributed under the MIT Software License
+# See accompanying file LICENSE.txt or copy at
+# https://opensource.org/licenses/MIT
+#
+# The documentation can be found at the library's page:
+# https://github.com/onqtam/ucm
+
+cmake_minimum_required(VERSION 2.8.12)
+
+include(CMakeParseArguments)
+
+# optionally include cotire - the git submodule might not be inited (or the user might have already included it)
+if(NOT COMMAND cotire)
+ include(${CMAKE_CURRENT_LIST_DIR}/../cotire/CMake/cotire.cmake OPTIONAL)
+endif()
+
+if(COMMAND cotire AND "1.7.9" VERSION_LESS "${COTIRE_CMAKE_MODULE_VERSION}")
+ set(ucm_with_cotire 1)
+else()
+ set(ucm_with_cotire 0)
+endif()
+
+option(UCM_UNITY_BUILD "Enable unity build for targets registered with the ucm_add_target() macro" OFF)
+option(UCM_NO_COTIRE_FOLDER "Do not use a cotire folder in the solution explorer for all unity and cotire related targets" ON)
+
+# ucm_add_flags
+# Adds compiler flags to CMAKE__FLAGS or to a specific config
+macro(ucm_add_flags)
+ cmake_parse_arguments(ARG "C;CXX;CLEAR_OLD" "" "CONFIG" ${ARGN})
+
+ if(NOT ARG_CONFIG)
+ set(ARG_CONFIG " ")
+ endif()
+
+ foreach(CONFIG ${ARG_CONFIG})
+ # determine to which flags to add
+ if(NOT ${CONFIG} STREQUAL " ")
+ string(TOUPPER ${CONFIG} CONFIG)
+ set(CXX_FLAGS CMAKE_CXX_FLAGS_${CONFIG})
+ set(C_FLAGS CMAKE_C_FLAGS_${CONFIG})
+ else()
+ set(CXX_FLAGS CMAKE_CXX_FLAGS)
+ set(C_FLAGS CMAKE_C_FLAGS)
+ endif()
+
+ # clear the old flags
+ if(${ARG_CLEAR_OLD})
+ if("${ARG_CXX}" OR NOT "${ARG_C}")
+ set(${CXX_FLAGS} "")
+ endif()
+ if("${ARG_C}" OR NOT "${ARG_CXX}")
+ set(${C_FLAGS} "")
+ endif()
+ endif()
+
+ # add all the passed flags
+ foreach(flag ${ARG_UNPARSED_ARGUMENTS})
+ if("${ARG_CXX}" OR NOT "${ARG_C}")
+ set(${CXX_FLAGS} "${${CXX_FLAGS}} ${flag}")
+ endif()
+ if("${ARG_C}" OR NOT "${ARG_CXX}")
+ set(${C_FLAGS} "${${C_FLAGS}} ${flag}")
+ endif()
+ endforeach()
+ endforeach()
+
+endmacro()
+
+# ucm_set_flags
+# Sets the CMAKE__FLAGS compiler flags or for a specific config
+macro(ucm_set_flags)
+ ucm_add_flags(CLEAR_OLD ${ARGN})
+endmacro()
+
+# ucm_add_linker_flags
+# Adds linker flags to CMAKE__LINKER_FLAGS or to a specific config
+macro(ucm_add_linker_flags)
+ cmake_parse_arguments(ARG "CLEAR_OLD;EXE;MODULE;SHARED;STATIC" "" "CONFIG" ${ARGN})
+
+ if(NOT ARG_CONFIG)
+ set(ARG_CONFIG " ")
+ endif()
+
+ foreach(CONFIG ${ARG_CONFIG})
+ string(TOUPPER "${CONFIG}" CONFIG)
+
+ if(NOT ${ARG_EXE} AND NOT ${ARG_MODULE} AND NOT ${ARG_SHARED} AND NOT ${ARG_STATIC})
+ set(ARG_EXE 1)
+ set(ARG_MODULE 1)
+ set(ARG_SHARED 1)
+ set(ARG_STATIC 1)
+ endif()
+
+ set(flags_configs "")
+ if(${ARG_EXE})
+ if(NOT "${CONFIG}" STREQUAL " ")
+ list(APPEND flags_configs CMAKE_EXE_LINKER_FLAGS_${CONFIG})
+ else()
+ list(APPEND flags_configs CMAKE_EXE_LINKER_FLAGS)
+ endif()
+ endif()
+ if(${ARG_MODULE})
+ if(NOT "${CONFIG}" STREQUAL " ")
+ list(APPEND flags_configs CMAKE_MODULE_LINKER_FLAGS_${CONFIG})
+ else()
+ list(APPEND flags_configs CMAKE_MODULE_LINKER_FLAGS)
+ endif()
+ endif()
+ if(${ARG_SHARED})
+ if(NOT "${CONFIG}" STREQUAL " ")
+ list(APPEND flags_configs CMAKE_SHARED_LINKER_FLAGS_${CONFIG})
+ else()
+ list(APPEND flags_configs CMAKE_SHARED_LINKER_FLAGS)
+ endif()
+ endif()
+ if(${ARG_STATIC})
+ if(NOT "${CONFIG}" STREQUAL " ")
+ list(APPEND flags_configs CMAKE_STATIC_LINKER_FLAGS_${CONFIG})
+ else()
+ list(APPEND flags_configs CMAKE_STATIC_LINKER_FLAGS)
+ endif()
+ endif()
+
+ # clear the old flags
+ if(${ARG_CLEAR_OLD})
+ foreach(flags ${flags_configs})
+ set(${flags} "")
+ endforeach()
+ endif()
+
+ # add all the passed flags
+ foreach(flag ${ARG_UNPARSED_ARGUMENTS})
+ foreach(flags ${flags_configs})
+ set(${flags} "${${flags}} ${flag}")
+ endforeach()
+ endforeach()
+ endforeach()
+endmacro()
+
+# ucm_set_linker_flags
+# Sets the CMAKE__LINKER_FLAGS linker flags or for a specific config
+macro(ucm_set_linker_flags)
+ ucm_add_linker_flags(CLEAR_OLD ${ARGN})
+endmacro()
+
+# ucm_gather_flags
+# Gathers all lists of flags for printing or manipulation
+macro(ucm_gather_flags with_linker result)
+ set(${result} "")
+ # add the main flags without a config
+ list(APPEND ${result} CMAKE_C_FLAGS)
+ list(APPEND ${result} CMAKE_CXX_FLAGS)
+ if(${with_linker})
+ list(APPEND ${result} CMAKE_EXE_LINKER_FLAGS)
+ list(APPEND ${result} CMAKE_MODULE_LINKER_FLAGS)
+ list(APPEND ${result} CMAKE_SHARED_LINKER_FLAGS)
+ list(APPEND ${result} CMAKE_STATIC_LINKER_FLAGS)
+ endif()
+
+ if("${CMAKE_CONFIGURATION_TYPES}" STREQUAL "" AND NOT "${CMAKE_BUILD_TYPE}" STREQUAL "")
+ # handle single config generators - like makefiles/ninja - when CMAKE_BUILD_TYPE is set
+ string(TOUPPER ${CMAKE_BUILD_TYPE} config)
+ list(APPEND ${result} CMAKE_C_FLAGS_${config})
+ list(APPEND ${result} CMAKE_CXX_FLAGS_${config})
+ if(${with_linker})
+ list(APPEND ${result} CMAKE_EXE_LINKER_FLAGS_${config})
+ list(APPEND ${result} CMAKE_MODULE_LINKER_FLAGS_${config})
+ list(APPEND ${result} CMAKE_SHARED_LINKER_FLAGS_${config})
+ list(APPEND ${result} CMAKE_STATIC_LINKER_FLAGS_${config})
+ endif()
+ else()
+ # handle multi config generators (like msvc, xcode)
+ foreach(config ${CMAKE_CONFIGURATION_TYPES})
+ string(TOUPPER ${config} config)
+ list(APPEND ${result} CMAKE_C_FLAGS_${config})
+ list(APPEND ${result} CMAKE_CXX_FLAGS_${config})
+ if(${with_linker})
+ list(APPEND ${result} CMAKE_EXE_LINKER_FLAGS_${config})
+ list(APPEND ${result} CMAKE_MODULE_LINKER_FLAGS_${config})
+ list(APPEND ${result} CMAKE_SHARED_LINKER_FLAGS_${config})
+ list(APPEND ${result} CMAKE_STATIC_LINKER_FLAGS_${config})
+ endif()
+ endforeach()
+ endif()
+endmacro()
+
+# ucm_set_runtime
+# Sets the runtime (static/dynamic) for msvc/gcc
+macro(ucm_set_runtime)
+ cmake_parse_arguments(ARG "STATIC;DYNAMIC" "" "" ${ARGN})
+
+ if(ARG_UNPARSED_ARGUMENTS)
+ message(FATAL_ERROR "unrecognized arguments: ${ARG_UNPARSED_ARGUMENTS}")
+ endif()
+
+ if(CMAKE_CXX_COMPILER_ID MATCHES "Clang" STREQUAL "")
+ message(AUTHOR_WARNING "ucm_set_runtime() does not support clang yet!")
+ endif()
+
+ ucm_gather_flags(0 flags_configs)
+
+ # add/replace the flags
+ # note that if the user has messed with the flags directly this function might fail
+ # - for example if with MSVC and the user has removed the flags - here we just switch/replace them
+ if("${ARG_STATIC}")
+ foreach(flags ${flags_configs})
+ if(CMAKE_CXX_COMPILER_ID MATCHES "GNU")
+ if(NOT ${flags} MATCHES "-static-libstdc\\+\\+")
+ set(${flags} "${${flags}} -static-libstdc++")
+ endif()
+ if(NOT ${flags} MATCHES "-static-libgcc")
+ set(${flags} "${${flags}} -static-libgcc")
+ endif()
+ elseif(MSVC)
+ if(${flags} MATCHES "/MD")
+ string(REGEX REPLACE "/MD" "/MT" ${flags} "${${flags}}")
+ endif()
+ endif()
+ endforeach()
+ elseif("${ARG_DYNAMIC}")
+ foreach(flags ${flags_configs})
+ if(CMAKE_CXX_COMPILER_ID MATCHES "GNU")
+ if(${flags} MATCHES "-static-libstdc\\+\\+")
+ string(REGEX REPLACE "-static-libstdc\\+\\+" "" ${flags} "${${flags}}")
+ endif()
+ if(${flags} MATCHES "-static-libgcc")
+ string(REGEX REPLACE "-static-libgcc" "" ${flags} "${${flags}}")
+ endif()
+ elseif(MSVC)
+ if(${flags} MATCHES "/MT")
+ string(REGEX REPLACE "/MT" "/MD" ${flags} "${${flags}}")
+ endif()
+ endif()
+ endforeach()
+ endif()
+endmacro()
+
+# ucm_print_flags
+# Prints all compiler flags for all configurations
+macro(ucm_print_flags)
+ ucm_gather_flags(1 flags_configs)
+ message("")
+ foreach(flags ${flags_configs})
+ message("${flags}: ${${flags}}")
+ endforeach()
+ message("")
+endmacro()
+
+# ucm_set_xcode_attrib
+# Set xcode attributes - name value CONFIG config1 conifg2..
+macro(ucm_set_xcode_attrib)
+ cmake_parse_arguments(ARG "" "CLEAR" "CONFIG" ${ARGN})
+
+ if(NOT ARG_CONFIG)
+ set(ARG_CONFIG " ")
+ endif()
+
+ foreach(CONFIG ${ARG_CONFIG})
+ # determine to which attributes to add
+ if(${CONFIG} STREQUAL " ")
+ if(${ARG_CLEAR})
+ # clear the old flags
+ unset(CMAKE_XCODE_ATTRIBUTE_${ARGV0})
+ else()
+ set(CMAKE_XCODE_ATTRIBUTE_${ARGV0} ${ARGV1})
+ endif()
+ else()
+ if(${ARG_CLEAR})
+ # clear the old flags
+ unset(CMAKE_XCODE_ATTRIBUTE_${ARGV0}[variant=${CONFIG}])
+ else()
+ set(CMAKE_XCODE_ATTRIBUTE_${ARGV0}[variant=${CONFIG}] ${ARGV1})
+ endif()
+ endif()
+ endforeach()
+endmacro()
+
+# ucm_count_sources
+# Counts the number of source files
+macro(ucm_count_sources)
+ cmake_parse_arguments(ARG "" "RESULT" "" ${ARGN})
+ if(${ARG_RESULT} STREQUAL "")
+ message(FATAL_ERROR "Need to pass RESULT and a variable name to ucm_count_sources()")
+ endif()
+
+ set(result 0)
+ foreach(SOURCE_FILE ${ARG_UNPARSED_ARGUMENTS})
+ if("${SOURCE_FILE}" MATCHES \\.\(c|C|cc|cp|cpp|CPP|c\\+\\+|cxx|i|ii\)$)
+ math(EXPR result "${result} + 1")
+ endif()
+ endforeach()
+ set(${ARG_RESULT} ${result})
+endmacro()
+
+# ucm_include_file_in_sources
+# Includes the file to the source with compiler flags
+macro(ucm_include_file_in_sources)
+ cmake_parse_arguments(ARG "" "HEADER" "" ${ARGN})
+ if(${ARG_HEADER} STREQUAL "")
+ message(FATAL_ERROR "Need to pass HEADER and a header file to ucm_include_file_in_sources()")
+ endif()
+
+ foreach(src ${ARG_UNPARSED_ARGUMENTS})
+ if(${src} MATCHES \\.\(c|C|cc|cp|cpp|CPP|c\\+\\+|cxx\)$)
+ # get old flags
+ get_source_file_property(old_compile_flags ${src} COMPILE_FLAGS)
+ if(old_compile_flags STREQUAL "NOTFOUND")
+ set(old_compile_flags "")
+ endif()
+
+ # update flags
+ if(MSVC)
+ set_source_files_properties(${src} PROPERTIES COMPILE_FLAGS
+ "${old_compile_flags} /FI\"${CMAKE_CURRENT_SOURCE_DIR}/${ARG_HEADER}\"")
+ else()
+ set_source_files_properties(${src} PROPERTIES COMPILE_FLAGS
+ "${old_compile_flags} -include \"${CMAKE_CURRENT_SOURCE_DIR}/${ARG_HEADER}\"")
+ endif()
+ endif()
+ endforeach()
+endmacro()
+
+# ucm_dir_list
+# Returns a list of subdirectories for a given directory
+macro(ucm_dir_list thedir result)
+ file(GLOB sub-dir "${thedir}/*")
+ set(list_of_dirs "")
+ foreach(dir ${sub-dir})
+ if(IS_DIRECTORY ${dir})
+ get_filename_component(DIRNAME ${dir} NAME)
+ LIST(APPEND list_of_dirs ${DIRNAME})
+ endif()
+ endforeach()
+ set(${result} ${list_of_dirs})
+endmacro()
+
+# ucm_trim_front_words
+# Trims X times the front word from a string separated with "/" and removes
+# the front "/" characters after that (used for filters for visual studio)
+macro(ucm_trim_front_words source out num_filter_trims)
+ set(result "${source}")
+ set(counter 0)
+ while(${counter} LESS ${num_filter_trims})
+ MATH(EXPR counter "${counter} + 1")
+ # removes everything at the front up to a "/" character
+ string(REGEX REPLACE "^([^/]+)" "" result "${result}")
+ # removes all consecutive "/" characters from the front
+ string(REGEX REPLACE "^(/+)" "" result "${result}")
+ endwhile()
+ set(${out} ${result})
+endmacro()
+
+# ucm_remove_files
+# Removes source files from a list of sources (path is the relative path for it to be found)
+macro(ucm_remove_files)
+ cmake_parse_arguments(ARG "" "FROM" "" ${ARGN})
+
+ if("${ARG_UNPARSED_ARGUMENTS}" STREQUAL "")
+ message(FATAL_ERROR "Need to pass some relative files to ucm_remove_files()")
+ endif()
+ if(${ARG_FROM} STREQUAL "")
+ message(FATAL_ERROR "Need to pass FROM and a variable name to ucm_remove_files()")
+ endif()
+
+ foreach(cur_file ${ARG_UNPARSED_ARGUMENTS})
+ list(REMOVE_ITEM ${ARG_FROM} ${cur_file})
+ endforeach()
+endmacro()
+
+# ucm_remove_directories
+# Removes all source files from the given directories from the sources list
+macro(ucm_remove_directories)
+ cmake_parse_arguments(ARG "" "FROM" "MATCHES" ${ARGN})
+
+ if("${ARG_UNPARSED_ARGUMENTS}" STREQUAL "")
+ message(FATAL_ERROR "Need to pass some relative directories to ucm_remove_directories()")
+ endif()
+ if(${ARG_FROM} STREQUAL "")
+ message(FATAL_ERROR "Need to pass FROM and a variable name to ucm_remove_directories()")
+ endif()
+
+ foreach(cur_dir ${ARG_UNPARSED_ARGUMENTS})
+ foreach(cur_file ${${ARG_FROM}})
+ string(REGEX MATCH ${cur_dir} res ${cur_file})
+ if(NOT "${res}" STREQUAL "")
+ if("${ARG_MATCHES}" STREQUAL "")
+ list(REMOVE_ITEM ${ARG_FROM} ${cur_file})
+ else()
+ foreach(curr_ptrn ${ARG_MATCHES})
+ string(REGEX MATCH ${curr_ptrn} res ${cur_file})
+ if(NOT "${res}" STREQUAL "")
+ list(REMOVE_ITEM ${ARG_FROM} ${cur_file})
+ break()
+ endif()
+ endforeach()
+ endif()
+ endif()
+ endforeach()
+ endforeach()
+endmacro()
+
+# ucm_add_files_impl
+macro(ucm_add_files_impl result trim files)
+ foreach(cur_file ${files})
+ SET(${result} ${${result}} ${cur_file})
+ get_filename_component(FILEPATH ${cur_file} PATH)
+ ucm_trim_front_words("${FILEPATH}" FILEPATH "${trim}")
+ # replacing forward slashes with back slashes so filters can be generated (back slash used in parsing...)
+ STRING(REPLACE "/" "\\" FILTERS "${FILEPATH}")
+ SOURCE_GROUP("${FILTERS}" FILES ${cur_file})
+ endforeach()
+endmacro()
+
+# ucm_add_files
+# Adds files to a list of sources
+macro(ucm_add_files)
+ cmake_parse_arguments(ARG "" "TO;FILTER_POP" "" ${ARGN})
+
+ if("${ARG_UNPARSED_ARGUMENTS}" STREQUAL "")
+ message(FATAL_ERROR "Need to pass some relative files to ucm_add_files()")
+ endif()
+ if(${ARG_TO} STREQUAL "")
+ message(FATAL_ERROR "Need to pass TO and a variable name to ucm_add_files()")
+ endif()
+
+ if("${ARG_FILTER_POP}" STREQUAL "")
+ set(ARG_FILTER_POP 0)
+ endif()
+
+ ucm_add_files_impl(${ARG_TO} ${ARG_FILTER_POP} "${ARG_UNPARSED_ARGUMENTS}")
+endmacro()
+
+# ucm_add_dir_impl
+macro(ucm_add_dir_impl result rec trim dirs_in additional_ext)
+ set(dirs "${dirs_in}")
+
+ # handle the "" and "." cases
+ if("${dirs}" STREQUAL "" OR "${dirs}" STREQUAL ".")
+ set(dirs "./")
+ endif()
+
+ foreach(cur_dir ${dirs})
+ # to circumvent some linux/cmake/path issues - barely made it work...
+ if(cur_dir STREQUAL "./")
+ set(cur_dir "")
+ else()
+ set(cur_dir "${cur_dir}/")
+ endif()
+
+ # since unix is case sensitive - add these valid extensions too
+ # we don't use "UNIX" but instead "CMAKE_HOST_UNIX" because we might be cross
+ # compiling (for example emscripten) under windows and UNIX may be set to 1
+ # Also OSX is case insensitive like windows...
+ set(additional_file_extensions "")
+ if(CMAKE_HOST_UNIX AND NOT APPLE)
+ set(additional_file_extensions
+ "${cur_dir}*.CPP"
+ "${cur_dir}*.C"
+ "${cur_dir}*.H"
+ "${cur_dir}*.HPP"
+ )
+ endif()
+
+ foreach(ext ${additional_ext})
+ list(APPEND additional_file_extensions "${cur_dir}*.${ext}")
+ endforeach()
+
+ # find all sources and set them as result
+ FILE(GLOB found_sources RELATIVE "${CMAKE_CURRENT_SOURCE_DIR}"
+ # https://gcc.gnu.org/onlinedocs/gcc-4.4.1/gcc/Overall-Options.html#index-file-name-suffix-71
+ # sources
+ "${cur_dir}*.cpp"
+ "${cur_dir}*.cxx"
+ "${cur_dir}*.c++"
+ "${cur_dir}*.cc"
+ "${cur_dir}*.cp"
+ "${cur_dir}*.c"
+ "${cur_dir}*.i"
+ "${cur_dir}*.ii"
+ # headers
+ "${cur_dir}*.h"
+ "${cur_dir}*.h++"
+ "${cur_dir}*.hpp"
+ "${cur_dir}*.hxx"
+ "${cur_dir}*.hh"
+ "${cur_dir}*.inl"
+ "${cur_dir}*.inc"
+ "${cur_dir}*.ipp"
+ "${cur_dir}*.ixx"
+ "${cur_dir}*.txx"
+ "${cur_dir}*.tpp"
+ "${cur_dir}*.tcc"
+ "${cur_dir}*.tpl"
+ ${additional_file_extensions})
+ SET(${result} ${${result}} ${found_sources})
+
+ # set the proper filters
+ ucm_trim_front_words("${cur_dir}" cur_dir "${trim}")
+ # replacing forward slashes with back slashes so filters can be generated (back slash used in parsing...)
+ STRING(REPLACE "/" "\\" FILTERS "${cur_dir}")
+ SOURCE_GROUP("${FILTERS}" FILES ${found_sources})
+ endforeach()
+
+ if(${rec})
+ foreach(cur_dir ${dirs})
+ ucm_dir_list("${cur_dir}" subdirs)
+ foreach(subdir ${subdirs})
+ ucm_add_dir_impl(${result} ${rec} ${trim} "${cur_dir}/${subdir}" "${additional_ext}")
+ endforeach()
+ endforeach()
+ endif()
+endmacro()
+
+# ucm_add_dirs
+# Adds all files from directories traversing them recursively to a list of sources
+# and generates filters according to their location (accepts relative paths only).
+# Also this macro trims X times the front word from the filter string for visual studio filters.
+macro(ucm_add_dirs)
+ cmake_parse_arguments(ARG "RECURSIVE" "TO;FILTER_POP" "ADDITIONAL_EXT" ${ARGN})
+
+ if(${ARG_TO} STREQUAL "")
+ message(FATAL_ERROR "Need to pass TO and a variable name to ucm_add_dirs()")
+ endif()
+
+ if("${ARG_FILTER_POP}" STREQUAL "")
+ set(ARG_FILTER_POP 0)
+ endif()
+
+ ucm_add_dir_impl(${ARG_TO} ${ARG_RECURSIVE} ${ARG_FILTER_POP} "${ARG_UNPARSED_ARGUMENTS}" "${ARG_ADDITIONAL_EXT}")
+endmacro()
+
+# ucm_add_target
+# Adds a target eligible for cotiring - unity build and/or precompiled header
+macro(ucm_add_target)
+ cmake_parse_arguments(ARG "UNITY" "NAME;TYPE;PCH_FILE;CPP_PER_UNITY" "UNITY_EXCLUDED;SOURCES" ${ARGN})
+
+ if(NOT "${ARG_UNPARSED_ARGUMENTS}" STREQUAL "")
+ message(FATAL_ERROR "Unrecognized options passed to ucm_add_target()")
+ endif()
+ if("${ARG_NAME}" STREQUAL "")
+ message(FATAL_ERROR "Need to pass NAME and a name for the target to ucm_add_target()")
+ endif()
+ set(valid_types EXECUTABLE STATIC SHARED MODULE)
+ list(FIND valid_types "${ARG_TYPE}" is_type_valid)
+ if(${is_type_valid} STREQUAL "-1")
+ message(FATAL_ERROR "Need to pass TYPE and the type for the target [EXECUTABLE/STATIC/SHARED/MODULE] to ucm_add_target()")
+ endif()
+ if("${ARG_SOURCES}" STREQUAL "")
+ message(FATAL_ERROR "Need to pass SOURCES and a list of source files to ucm_add_target()")
+ endif()
+
+ # init with the global unity flag
+ set(do_unity ${UCM_UNITY_BUILD})
+
+ # check the UNITY argument
+ if(NOT ARG_UNITY)
+ set(do_unity FALSE)
+ endif()
+
+ # if target is excluded through the exclusion list
+ list(FIND UCM_UNITY_BUILD_EXCLUDE_TARGETS ${ARG_NAME} is_target_excluded)
+ if(NOT ${is_target_excluded} STREQUAL "-1")
+ set(do_unity FALSE)
+ endif()
+
+ # unity build only for targets with > 1 source file (otherwise there will be an additional unnecessary target)
+ if(do_unity) # optimization
+ ucm_count_sources(${ARG_SOURCES} RESULT num_sources)
+ if(${num_sources} LESS 2)
+ set(do_unity FALSE)
+ endif()
+ endif()
+
+ set(wanted_cotire ${do_unity})
+
+ # if cotire cannot be used
+ if(do_unity AND NOT ucm_with_cotire)
+ set(do_unity FALSE)
+ endif()
+
+ # inform the developer that the current target might benefit from a unity build
+ if(NOT ARG_UNITY AND ${UCM_UNITY_BUILD})
+ ucm_count_sources(${ARG_SOURCES} RESULT num_sources)
+ if(${num_sources} GREATER 1)
+ message(AUTHOR_WARNING "Target '${ARG_NAME}' may benefit from a unity build.\nIt has ${num_sources} sources - enable with UNITY flag")
+ endif()
+ endif()
+
+ # prepare for the unity build
+ set(orig_target ${ARG_NAME})
+ if(do_unity)
+ # the original target will be added with a different name than the requested
+ set(orig_target ${ARG_NAME}_ORIGINAL)
+
+ # exclude requested files from unity build of the current target
+ foreach(excluded_file "${ARG_UNITY_EXCLUDED}")
+ set_source_files_properties(${excluded_file} PROPERTIES COTIRE_EXCLUDED TRUE)
+ endforeach()
+ endif()
+
+ # add the original target
+ if(${ARG_TYPE} STREQUAL "EXECUTABLE")
+ add_executable(${orig_target} ${ARG_SOURCES})
+ else()
+ add_library(${orig_target} ${ARG_TYPE} ${ARG_SOURCES})
+ endif()
+
+ if(do_unity)
+ # set the number of unity cpp files to be used for the unity target
+ if(NOT "${ARG_CPP_PER_UNITY}" STREQUAL "")
+ set_property(TARGET ${orig_target} PROPERTY COTIRE_UNITY_SOURCE_MAXIMUM_NUMBER_OF_INCLUDES "${ARG_CPP_PER_UNITY}")
+ else()
+ set_property(TARGET ${orig_target} PROPERTY COTIRE_UNITY_SOURCE_MAXIMUM_NUMBER_OF_INCLUDES "100")
+ endif()
+
+ if(NOT "${ARG_PCH_FILE}" STREQUAL "")
+ set_target_properties(${orig_target} PROPERTIES COTIRE_CXX_PREFIX_HEADER_INIT "${ARG_PCH_FILE}")
+ else()
+ set_target_properties(${orig_target} PROPERTIES COTIRE_ENABLE_PRECOMPILED_HEADER FALSE)
+ endif()
+ # add a unity target for the original one with the name intended for the original
+ set_target_properties(${orig_target} PROPERTIES COTIRE_UNITY_TARGET_NAME ${ARG_NAME})
+
+ # this is the library call that does the magic
+ cotire(${orig_target})
+ set_target_properties(clean_cotire PROPERTIES FOLDER "CMakePredefinedTargets")
+
+ # disable the original target and enable the unity one
+ get_target_property(unity_target_name ${orig_target} COTIRE_UNITY_TARGET_NAME)
+ set_target_properties(${orig_target} PROPERTIES EXCLUDE_FROM_ALL 1 EXCLUDE_FROM_DEFAULT_BUILD 1)
+ set_target_properties(${unity_target_name} PROPERTIES EXCLUDE_FROM_ALL 0 EXCLUDE_FROM_DEFAULT_BUILD 0)
+
+ # also set the name of the target output as the original one
+ set_target_properties(${unity_target_name} PROPERTIES OUTPUT_NAME ${ARG_NAME})
+ if(UCM_NO_COTIRE_FOLDER)
+ # reset the folder property so all unity targets dont end up in a single folder in the solution explorer of VS
+ set_target_properties(${unity_target_name} PROPERTIES FOLDER "")
+ endif()
+ set_target_properties(all_unity PROPERTIES FOLDER "CMakePredefinedTargets")
+ elseif(NOT "${ARG_PCH_FILE}" STREQUAL "")
+ set(wanted_cotire TRUE)
+ if(ucm_with_cotire)
+ set_target_properties(${orig_target} PROPERTIES COTIRE_ADD_UNITY_BUILD FALSE)
+ set_target_properties(${orig_target} PROPERTIES COTIRE_CXX_PREFIX_HEADER_INIT "${ARG_PCH_FILE}")
+ cotire(${orig_target})
+ set_target_properties(clean_cotire PROPERTIES FOLDER "CMakePredefinedTargets")
+ endif()
+ endif()
+
+ # print a message if the target was requested to be cotired but it couldn't
+ if(wanted_cotire AND NOT ucm_with_cotire)
+ if(NOT COMMAND cotire)
+ message(AUTHOR_WARNING "Target \"${ARG_NAME}\" not cotired because cotire isn't loaded")
+ else()
+ message(AUTHOR_WARNING "Target \"${ARG_NAME}\" not cotired because cotire is older than the required version")
+ endif()
+ endif()
+endmacro()
diff --git a/libs/sqlite_orm-1.8.2/dependencies/CMakeLists.txt b/libs/sqlite_orm-1.8.2/dependencies/CMakeLists.txt
new file mode 100644
index 0000000..2822439
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/dependencies/CMakeLists.txt
@@ -0,0 +1,28 @@
+include(FetchContent)
+
+if(CMAKE_PROJECT_NAME STREQUAL PROJECT_NAME AND BUILD_TESTING)
+ # FIND_PACKAGE_ARGS is available since 3.24
+ if(CMAKE_VERSION VERSION_LESS 3.24)
+ FetchContent_Declare(
+ Catch2
+ GIT_REPOSITORY https://github.com/catchorg/Catch2.git
+ GIT_TAG v3.2.1
+ )
+ else()
+ FetchContent_Declare(
+ Catch2
+ GIT_REPOSITORY https://github.com/catchorg/Catch2.git
+ GIT_TAG v3.2.1
+ # prefer find_package() over building from source
+ FIND_PACKAGE_ARGS 3 CONFIG
+ )
+ endif()
+endif()
+
+if(CMAKE_PROJECT_NAME STREQUAL PROJECT_NAME AND BUILD_TESTING)
+ # CMake <3.24: exposes targets only locally, but caches them. So call FetchContent_MakeAvailable again in the directory of usage
+ FetchContent_MakeAvailable(Catch2)
+endif()
+
+# CMake <3.24: exposes targets only locally, but caches them. So call find_package again in the directory of usage
+find_package(SQLite3 REQUIRED)
diff --git a/libs/sqlite_orm-1.8.2/dev/alias.h b/libs/sqlite_orm-1.8.2/dev/alias.h
new file mode 100644
index 0000000..eb5aad0
--- /dev/null
+++ b/libs/sqlite_orm-1.8.2/dev/alias.h
@@ -0,0 +1,196 @@
+#pragma once
+
+#include // std::enable_if, std::is_base_of, std::is_member_pointer, std::remove_const
+#include // std::index_sequence, std::make_index_sequence
+#include // std::string
+#include // std::stringstream
+#include // std::copy_n
+
+#include "functional/cxx_universal.h" // ::size_t
+#include "functional/cxx_type_traits_polyfill.h"
+#include "type_traits.h"
+#include "alias_traits.h"
+
+namespace sqlite_orm {
+
+ namespace internal {
+
+ /**
+ * This is a common built-in class used for character based table aliases.
+ * For convenience there exist public type aliases `alias_a`, `alias_b`, ...
+ * The easiest way to create a table alias is using `"z"_alias.for_